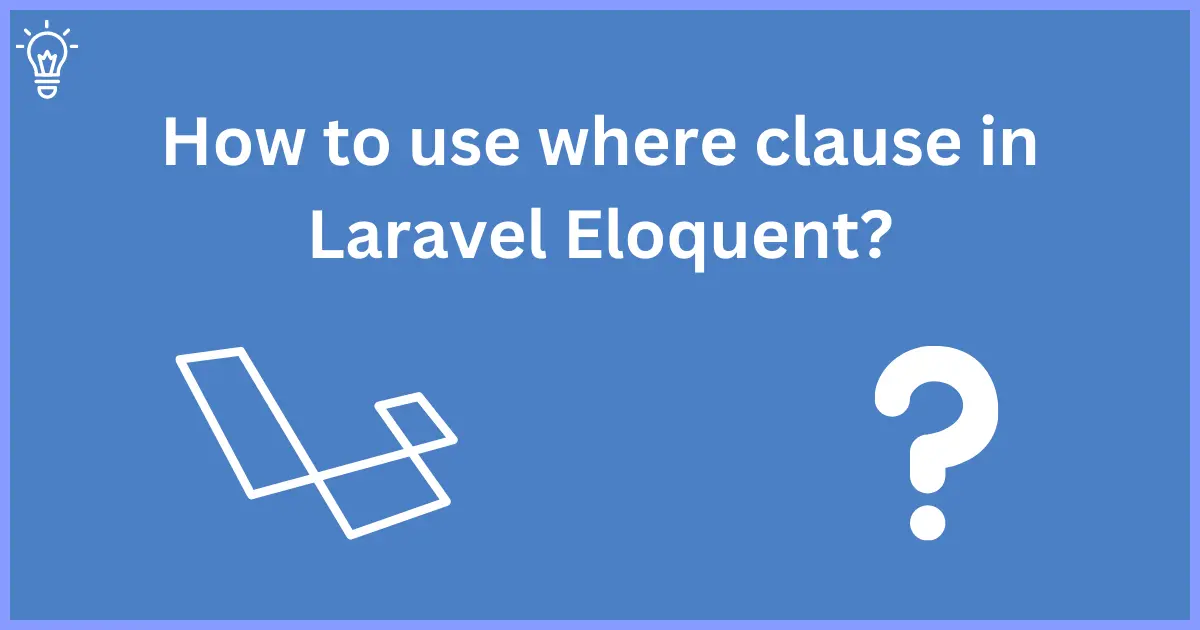
How to use where clause in Laravel Eloquent?
Hello Artisans.
Today, I'm going to show you how to use where clause in Laravel Eloquent? You will learn how to use multiple where conditions in Laravel eloquent. This tutorial goes into details on Laravel multiple where conditions in Eloquent query builder.
So, let's get started with examples.
Laravel multiple where conditions
Example 1: Simple Syntax
You can simply add multiple where()
function to add multiple where conditions in your Laravel eloquent query. So let's find the user with the name john wick and the email [email protected]
$user = User::where('name','John Wick')->where('email','[email protected]')->get();
Example 2: Array Syntax
By using array syntax you can add array in where function to add multiple where conditions.
$user = User::where([
['name','John Doe'],
['email','[email protected]']
])->first();
Raw SQL Query will be same for both of above methods.
Select * from users where name="John Wick" AND email="[email protected]";
By default, the where clause is chaining with the AND operator. To use OR operator in where clause you have to use orWhere()
function that we will discuss in next examples.
Laravel multiple orWhere conditions
In some cases you need to join your where clause with OR operator instead of AND. In this case you can use orWhere()
method.
Example
$user = User::where('email','[email protected]')->orWhere('email','[email protected]')->get();
The above example means, find user whose email is [email protected] or [email protected]
The raw SQL Query will be look like this:
Select * from users where email="[email protected]" OR email="[email protected]";
Group Multiple AND-OR-AND where conditions
Some times you have to use group multiple AND-OR-AND conditions when you are dealing with complex queries. You can not use multiple AND-OR-AND conditions together directly. Remember, In SQL queries, we use parentheses to group such complex conditions. We could encapsulates the condition in parentheses like this:
Read also: Explained where clause uses in laravel with examples
Example
$users = User::where('status','active')->where(function($query) {
$query->where('email','[email protected]')
->orWhere('email','[email protected]');
})->get();
Raw SQL query will be look like this:
select * from `users` where status='active' and (email ="[email protected]" OR email="[email protected]");
I hope it will help you out.
Happy Coding :)