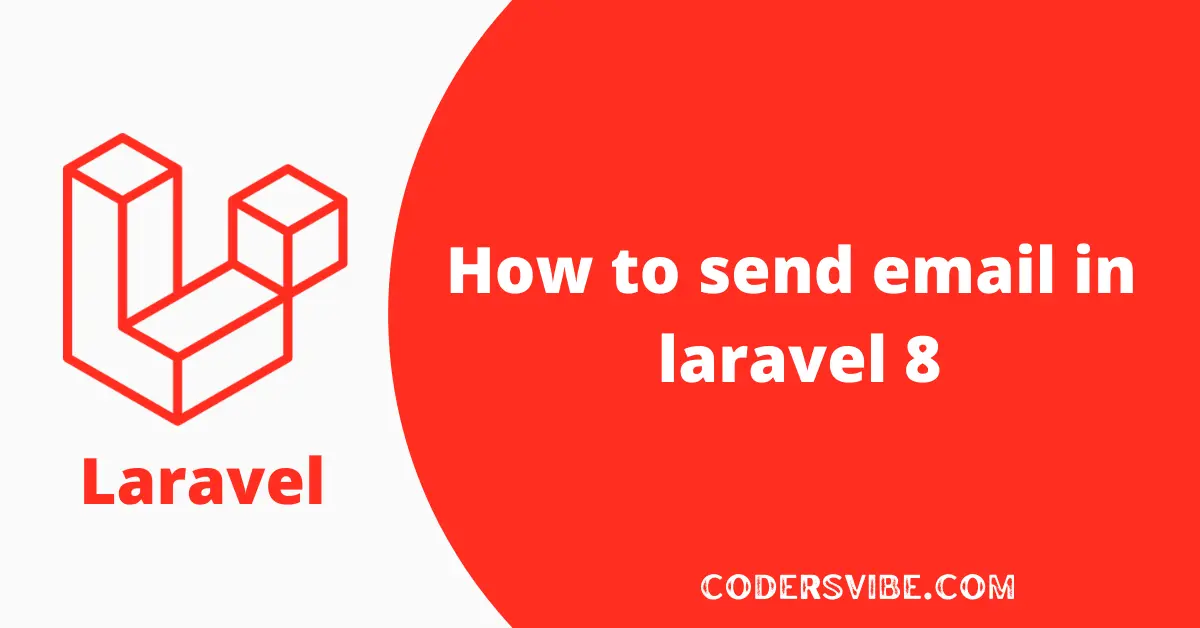
How to Send Email in Laravel 8?
Hello Artisans! In this article, We will discuss how to send email in Laravel with an example. Let's discuss How to send Emails in Laravel 8 in simple steps. This post will give you a simple and easy example of sending an email in Laravel 8 with SMTP. Let's follow the below step for sending mail in Laravel 8.
Laravel 8 provides a mail class to send an email. you can use several drivers to send email laravel 8. You can use SMTP, Mailgun, Postmark, Amazon SES, Send in Blue, and sendmail. You have to configure in the env file what driver you wanna use for your project.
In this tutorial, We will use Send in Blue provider. I will give you step by step guide to sending emails in laravel 8 with Send in Blue. You can create a blade file for your mail layout and also with dynamic information.
Step-1: Create your account on Send In Blue
In the first step, you have to create an account on Send In Blue and then create a new API Key.
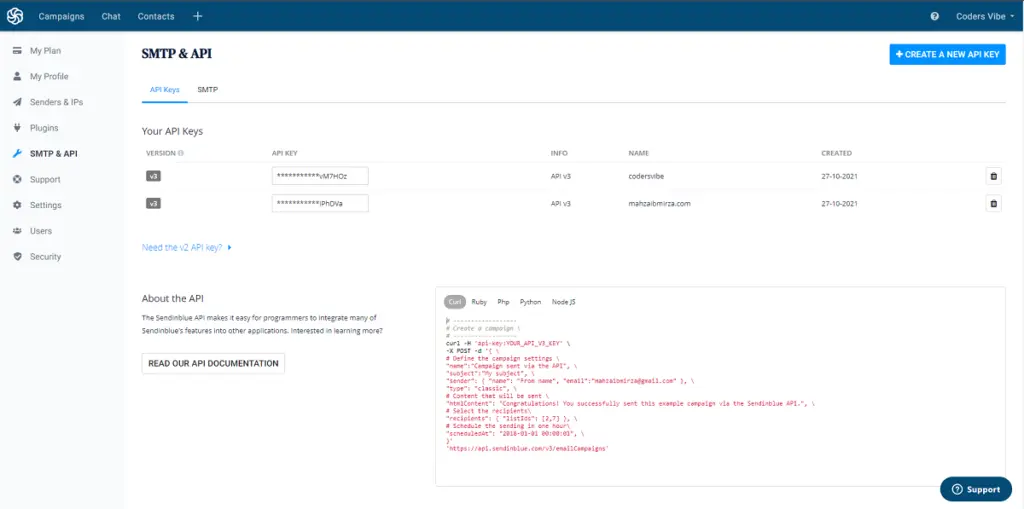
Step-2: Configure Send In Blue in your project
In the second step, you have to configure send in blue with mail driver, mail host, mail port, mail username, and mail password in your laravel project. So you have to edit the following code in your .env file.
...
MAIL_MAILER=smtp
MAIL_HOST=smtp-relay.sendinblue.com
MAIL_PORT=587
[email protected]
MAIL_PASSWORD=your-password
MAIL_ENCRYPTION=tls
[email protected]
MAIL_FROM_NAME="${APP_NAME}"
...
Step-3: Create Mail Class
In this step, we will create a mail class MyMail for email sending. To create a mail class just run the following command
php artisan make:mail MyMail
In this file, we will write code for which view should call and the object of the user.
<?php
namespace App\Mail;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Mail\Mailable;
use Illuminate\Queue\SerializesModels;
class MyMail extends Mailable
{
use Queueable, SerializesModels;
public $details;
/**
* Create a new message instance.
*
* @return void
*/
public function __construct($details)
{
$this->details = $details;
}
/**
* Build the message.
*
* @return $this
*/
public function build()
{
return $this->subject('Mail from Coders Vibe')
->view('mails.myMail');
}
}
Step-4: Create Blade view to design your email layout
In this step, we will create a blade file and design layout that we want to send. Now we just write some dummy text to show you as an example you can design as per your requirements. Create the below file view folder.
<!DOCTYPE html>
<html>
<head>
<title>Codersvibe.com</title>
</head>
<body>
<h1>{{ $details['title'] }}</h1>
<p>{{ $details['body'] }}</p>
<p>Thank you</p>
</body>
</html>
Step-5: Create Route in route/web.php file
Now at the end, we will create a route for sending our mail. So, let's create the below web route for testing and send an email.
...
Route::get('send-mail', function () {
$details = [
'title' => 'Mail from Codersvibe.com',
'body' => 'This is your first mail using smtp'
];
\Mail::to('[email protected]')->send(new \App\Mail\MyMail($details));
dd("Email is Sent.");
});
You can do it in a controller like this
<?php
namespace App\Http\Controllers;
use App\Mail\QueryMail;
use Illuminate\Support\Facades\Mail;
class MailController extends Controller
{
public function sendmail(){
$details = [
'title' => 'Mail from Codersvibe.com',
'body' => 'This is your first mail using smtp'
];
Mail::to('[email protected]')->send(new QueryMail($details));
dd('Mail Sent');
}
}
and then the route will be just in one line like this:
...
Route::get('send-mail', [MailController::class,'sendmail']);
Now you can run and check this example.
It will send your email, just run the following command and go to the following route.
Run Project:
php artisan serve
Open this link to send mail:
http://localhost:8000/send-mail
or
http://127.0.0.1:8000/send-mail
I hope it can be helpful to you
Happy Coding :)