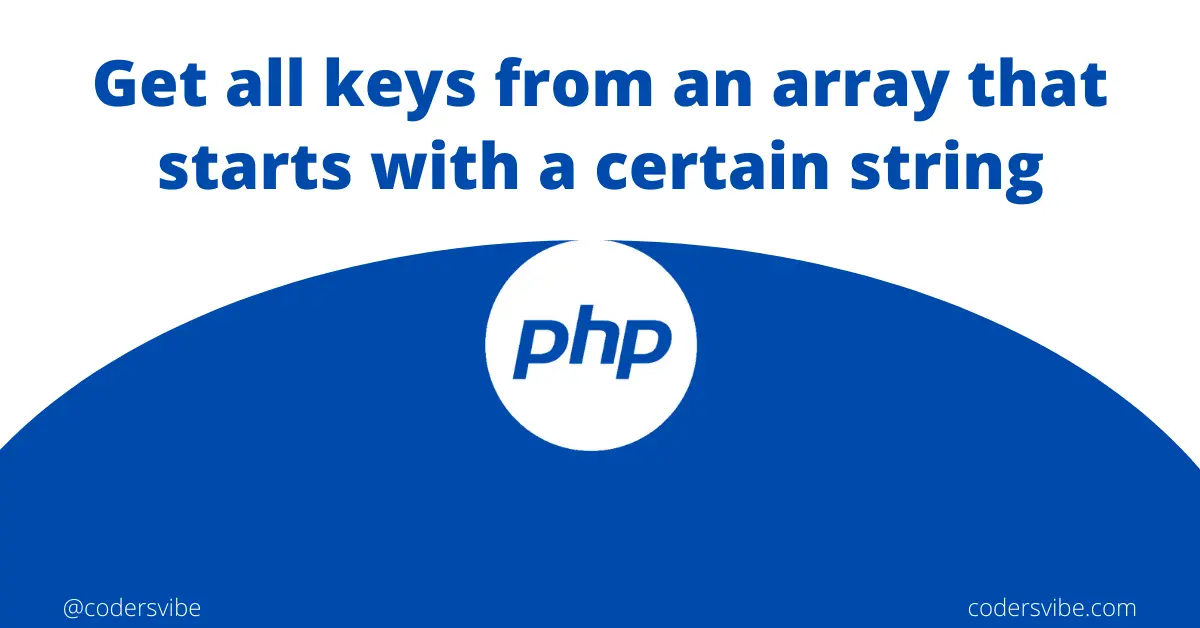
Get all keys from an array that starts with a certain string
Hello Devs!
In this post, I will show you how to get all keys from an array that starts with a certain string. I will show you a simple example of PHP array filtering only certain keys. I will help you to give some examples of getting all key-value starting with a certain string from a PHP array. It's gonna be a simple example of PHP array_filter_key() function. So, Let's see below examples of PHP array gets all keys to start with a specific string.
I will provide 4 examples here.
So, let's start one by one.
Example 1:
<?php
$arr = [
'fb-1' => 'One',
'fb-2' => 'Two',
'3' => 'Three',
'fb-4' => 'Four',
];
$result = [];
$startWith = 'fb';
foreach($arr as $key => $value){
$exp_key = explode('-', $key);
if($exp_key[0] == $startWith){
$result[] = $value;
}
}
print_r($result);
?>
Output:Array
(
[0] => One
[1] => Two
[2] => Four
)
Example 2:
It's gonna be a functional approach an array_filter_key()
the function is taken and applied to an array of elements.
<?php
$arr = [
'fb-1' => 'One',
'fb-2' => 'Two',
'3' => 'Three',
'fb-4' => 'Four',
];
$result = array_filter_key($arr, function($key) {
return strpos($key, 'fb-') === 0;
});
print_r($result);
?>
Output:
Array
(
[0] => One
[1] => Two
[2] => Four
)
Example 3:
In the third example, we will use a procedural approach.
<?php
$arr = [
'fb-1' => 'One',
'fb-2' => 'Two',
'3' => 'Three',
'fb-4' => 'Four',
];
$result = [];
foreach ($arr as $key => $value) {
if (strpos($key, 'fb-') === 0) {
$result[$key] = $value;
}
}
print_r($result);
?>
Output:
Array
(
[0] => One
[1] => Two
[2] => Four
)
Example 4:
In the last example, we will use a procedural approach but use objects.
<?php
$arr = [
'fb-1' => 'One',
'fb-2' => 'Two',
'3' => 'Three',
'fb-4' => 'Four',
];
$result = [];
$i = new ArrayIterator($arr);
while ($i->valid()) {
if (strpos($i->key(), 'fb-') === 0) {
$result[$i->key()] = $i->current();
}
$i->next();
}
print_r($result);
?>
Output:
Array
(
[0] => One
[1] => Two
[2] => Four
)
I hope it can be helpful.
Happy Coding :)