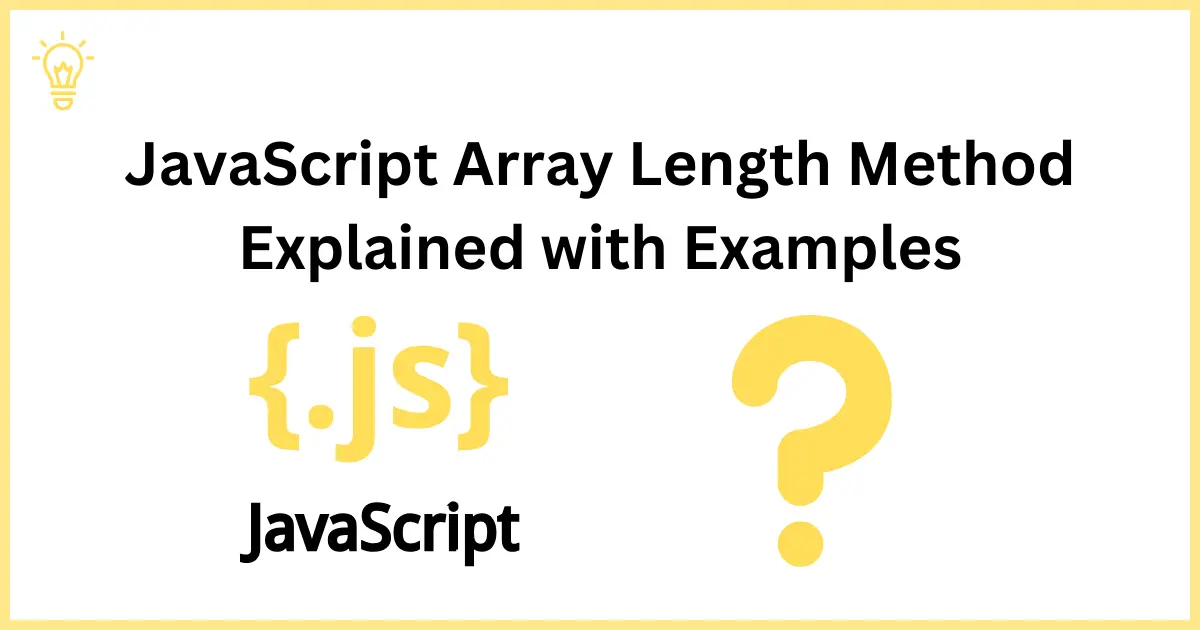
JavaScript Array Length Method Explained with Examples
For any developer, comprehension of JavaScript’s array is essential since it is a key player in the dynamic world. One vital element here is the ‘length’ function, which tells how many items are contained within an array. In this guide, we will explore the JavaScript Array Length method and explain its working principles by using practical examples.
How to find JavaScript array length?
Know three quick methods of finding the length of a JavaScript array: direct length
property, flexible for of
and for in
loops, and concise reduce()
function. Learn these strategies for fast determination of the length of an array in your JavaScript projects. You can use any of the following method according to your logic and scenario.
Using length() Property
The easiest and quick solution is to use length property to determine the array length in JavaScript. To get the length, simply append .length
to the array variable, and voila!
const myArray = [1, 2, 3, 4, 5];
const arrayLength = myArray.length;
console.log(arrayLength) // 5
Using for of and for in loop
If you don't wanna use length property to determine the size of array, you can use for of or for in loop methods. To find the length of a JavaScript array using the for of
and for in
loops, you can iterate through the array's elements.
Here's how you can use the for of
loop:
const myArray = [1, 2, 3, 4, 5];
let count = 0;
for (const element of myArray) {
count++;
}
console.log("Array length:", count); // 5
And here's the equivalent using the for in
loop:
const myArray = [1, 2, 3, 4, 5];
let count = 0;
for (const index in myArray) {
count++;
}
console.log("Array length:", count); // 5
Using reduce() function
Employing the reduce()
function is another concise way to determine the length of a JavaScript array. The reduce()
function iterates over each element of the array, accumulating a value based on a provided callback function. In this case, we can accumulate the count of elements. Here's how it looks:
const myArray = [1, 2, 3, 4, 5];
const arrayLength = myArray.reduce((acc) => acc + 1, 0);
console.log("Array length:", arrayLength);
Conclusion
In summary, understanding how to find the length of a JavaScript array using methods like length
property, for of
and for in
loops, and the reduce()
function is crucial. These versatile techniques offer diverse approaches for efficient array length retrieval, allowing developers to choose the method that best suits their coding preferences and project needs.
FAQs
Why is understanding JavaScript's array important for developers?
Comprehension of JavaScript's array is crucial for developers as it plays a key role in the dynamic world of programming.
What is the significance of the 'length' function in JavaScript arrays?
The 'length' function in JavaScript arrays is a vital element that indicates how many items are contained within an array.
How can I find the length of a JavaScript array quickly?
There are three quick methods to find the length of a JavaScript array: using the direct length property, employing for of and for in loops, and utilizing the concise reduce() function.
What is the easiest and quickest method to determine the array length in JavaScript?
The simplest and quickest method to determine the array length in JavaScript is by using the length property. Just append .length
to the array variable.
Can I find the array length without using the length property?
Yes, you can find the length of a JavaScript array without using the length property by using for of or for in loop methods, which involve iterating through the array's elements.