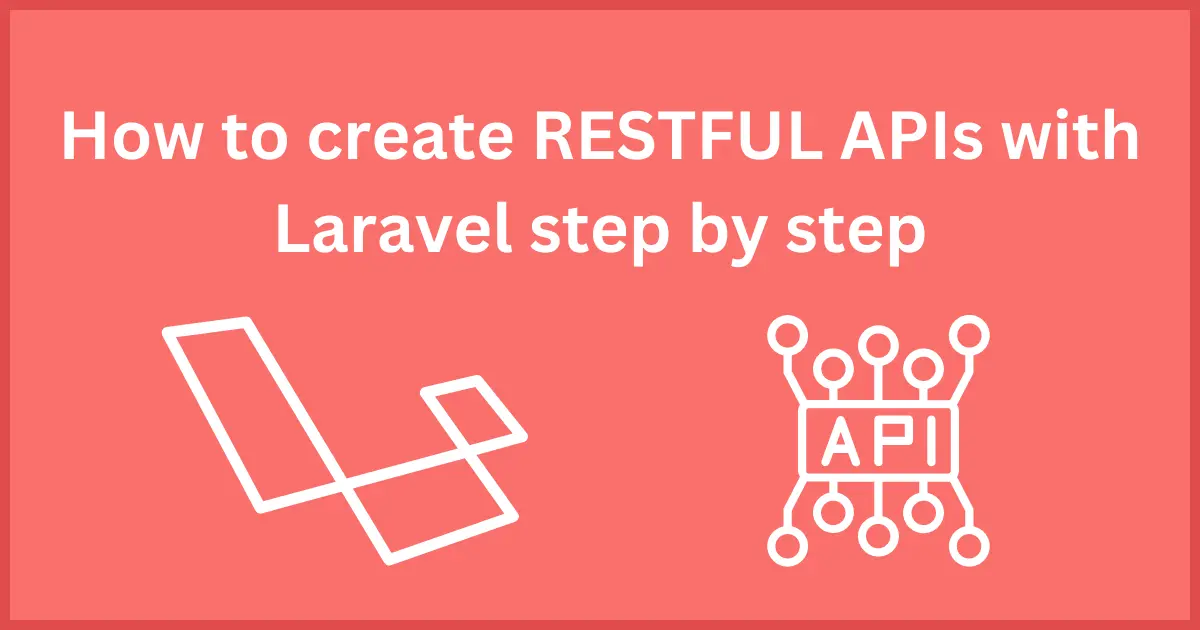
How to create RESTFUL APIs with Laravel step by step
Hello Artisans,
Today, I'm going to show you how to create a restful api with laravel step by step. We create laravel apis when we work with mobile applications. We can easily develop the APIs with Laravel, or hire Laravel developers to streamline the process and ensure optimal performance.
In this tutorial we will create a restful apis with laravel. So let's start the tutorial to create laravel rest apis.
Step 1: Install the Laravel
First of all, you need to install laravel application with the following command to create the rest apis. You can skip this step if you have already installed Laravel.
composer create-project laravel/laravel Laravel-Apis
You can specify the laravel version by following the command if you want to install any specific version of laravel.
composer create-project laravel/laravel:^8.0 Laravel-Apis
Step 2: Database Configuration
In the second step you need to configure the database in .env file to create the rest apis in laravel
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=database_name
DB_USERNAME=database_username
DB_PASSWORD=database_password
Step 3: Create a table
In this step you need to create a table to store data. Just run the following command to create table.
php artisan make:migration create_posts_table
Now update the migration file with the following code to add the columns in table.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
class CreatePostsTable extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('posts', function (Blueprint $table) {
$table->id();
$table->string('title');
$table->string('slug');
$table->text('body');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
}
Now you have to run the following command to migrate the table. I have added slug column also for SEO purposes.
php artisan migrate
Step 4: Create Api Routes
In this step you have to create api routes in your routes/api.php file. We will use resource routes to manage curd operation. Just add the following the code in your routes/api.php file.
use App\Http\Controllers\PostController;
Route::resource('posts', PostController::class);
Step 5: Create Controller & Model
Now you have to create controller and model to create the rest apis in laravel. Just run the following command in your terminal to create the post controller and model.
php artisan make:model Post -r
After running above command, you will find newly created files Post.php and PostController.php in Models and Controllers folder respectively.
In the controller, we have seven methods by default as given below that will be used for crud operation.
- index()
- create()
- store()
- show()
- edit()
- update()
- destroy()
But we don't have to use create() and edit() methods because these methods are used to show create and edit forms. So, now update the PostController file with the following code.
<?php
namespace App\Http\Controllers;
use App\Models\Post;
use Illuminate\Http\Request;
use Illuminate\Support\Str;
class PostController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
$posts = Post::latest()->paginate(10);
return [
"status" => 1,
"data" => $posts
];
}
/**
* Store a newly created resource in storage.
*
* @param \Illuminate\Http\Request $request
* @return \Illuminate\Http\Response
*/
public function store(Request $request)
{
$request->validate([
'title' => 'required',
'body' => 'required',
]);
$request['slug'] = Str::slug($request->title,'-');
$post = Post::create($request->all());
return [
"status" => 1,
"data" => $post
];
}
/**
* Display the specified resource.
*
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function show(Post $post)
{
return [
"status" => 1,
"data" =>$post
];
}
/**
* Update the specified resource in storage.
*
* @param \Illuminate\Http\Request $request
* @param \App\Models\Post $post
* @return \Illuminate\Http\Response
*/
public function update(Request $request, Post $post)
{
$request->validate([
'title' => 'required',
'body' => 'required',
]);
$request['slug'] = Str::slug($request->title,'-');
$post->update($request->all());
return [
"status" => 1,
"data" => $post,
"msg" => "Post updated successfully"
];
}
/**
* Remove the specified resource from storage.
*
* @param \App\Models\Blog $blog
* @return \Illuminate\Http\Response
*/
public function destroy(Post $post)
{
$post->delete();
return [
"status" => 1,
"data" => $post,
"msg" => "Post deleted successfully"
];
}
}
Step 6: Run the Application
Finally, you can start your application to test your rest apis. Just run the following command to run the laravel application.
php artisan serve
Step 7: Testing Rest APIs
We have successfully created Laravel restful apis. Now you can test these apis with Postman.
Create Post
url: http://127.0.0.1:8000/api/posts
method: POST
data: {title: “Post Title”, body: “Post Body”}
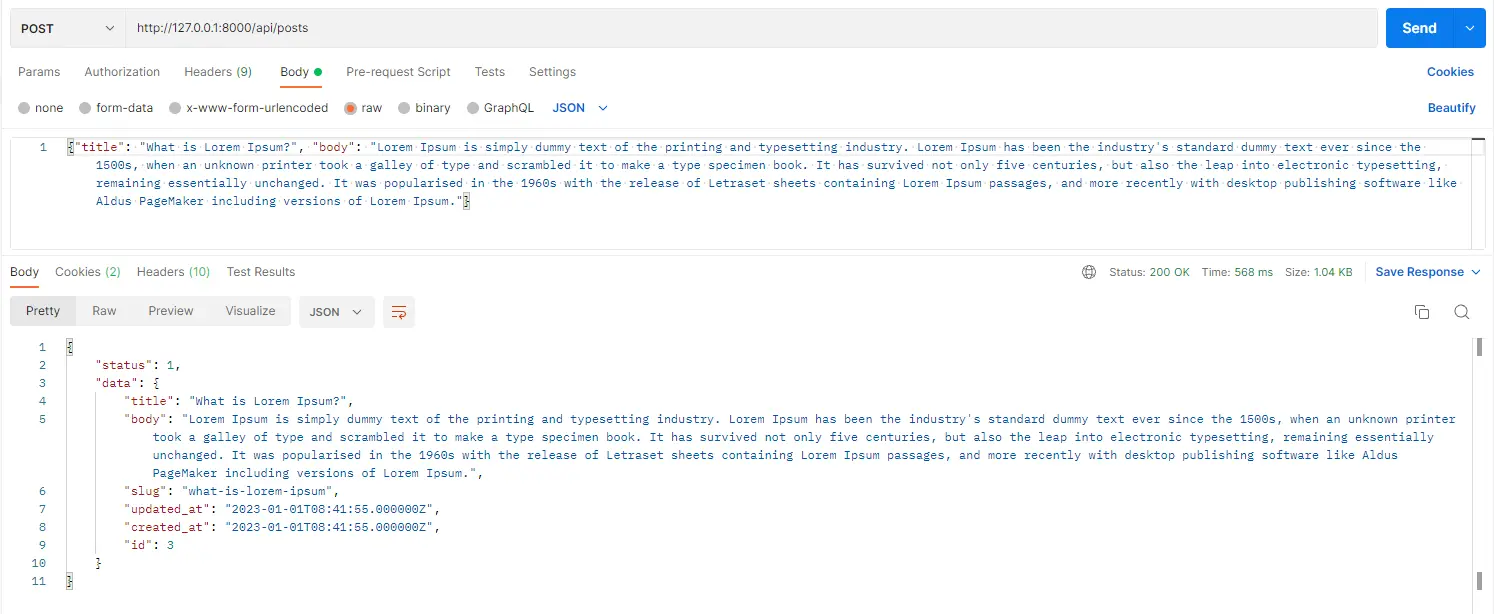
Update Post
url: http://127.0.0.1:8000/api/posts/{post-id}
method: PUT/PATCH
data: {title: “Update Post Title”, body: “Update Post Body”}
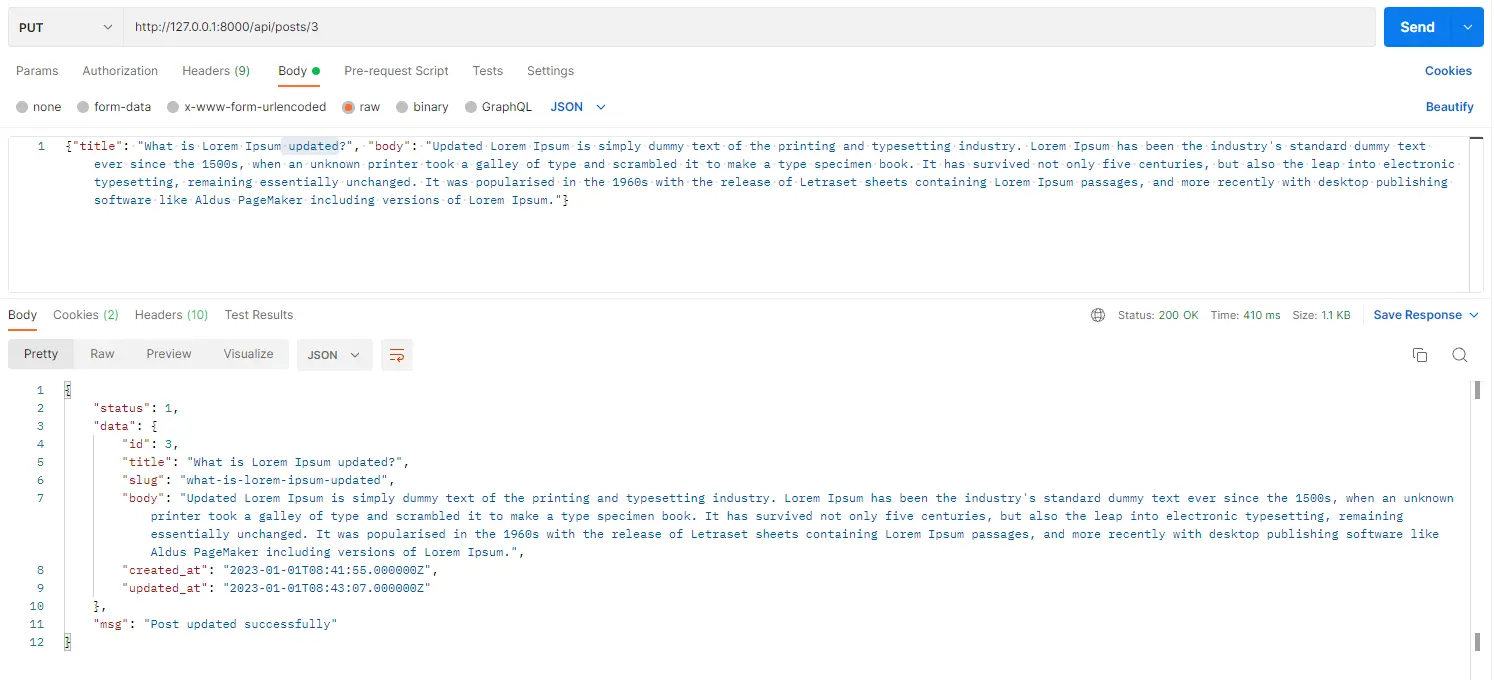
Get All Posts
url: http://127.0.0.1:8000/api/posts
method: GET
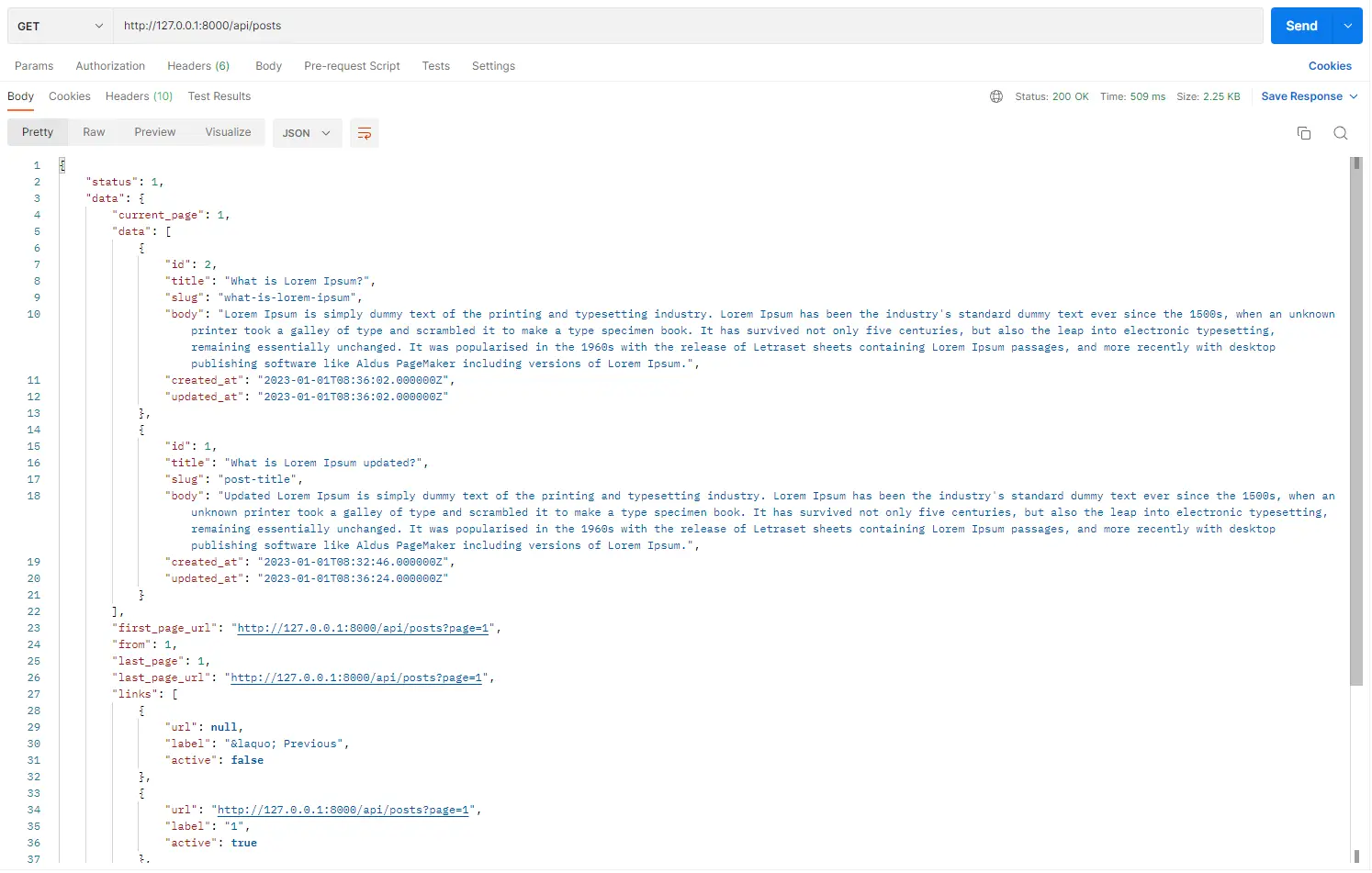
Get Single Post
url: http://127.0.0.1:8000/api/posts/{post-id}
method: GET
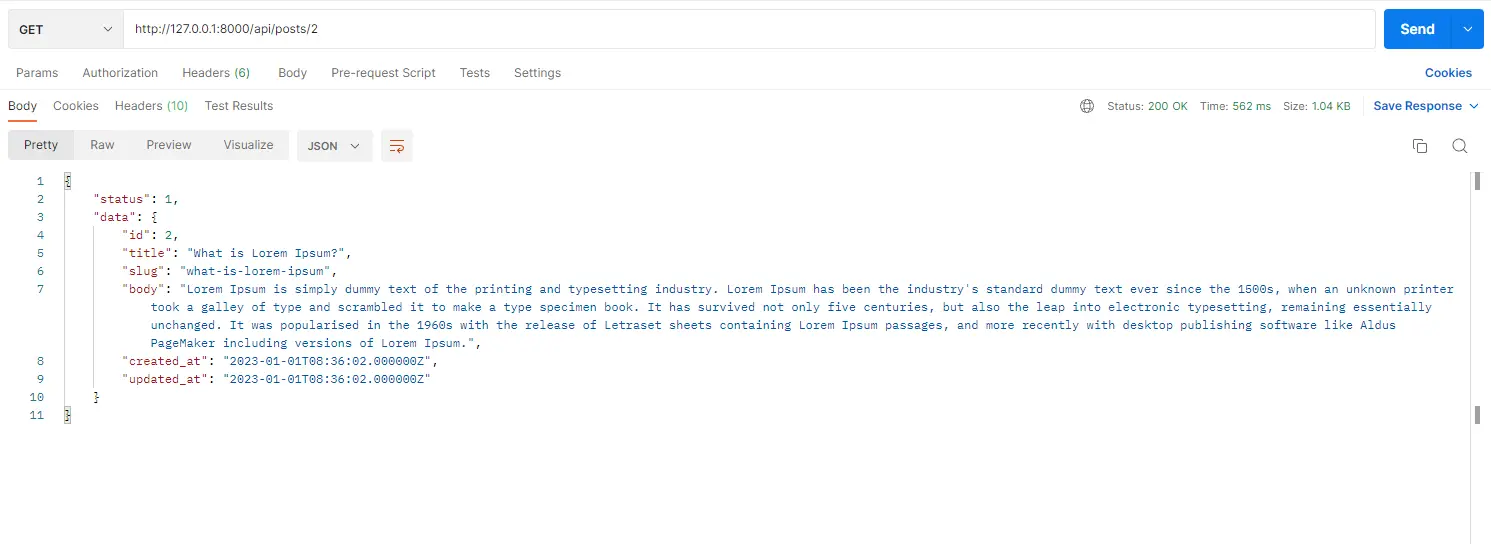
Delete Post
url: http://127.0.0.1:8000/api/posts/{post-id}
method: DELETE
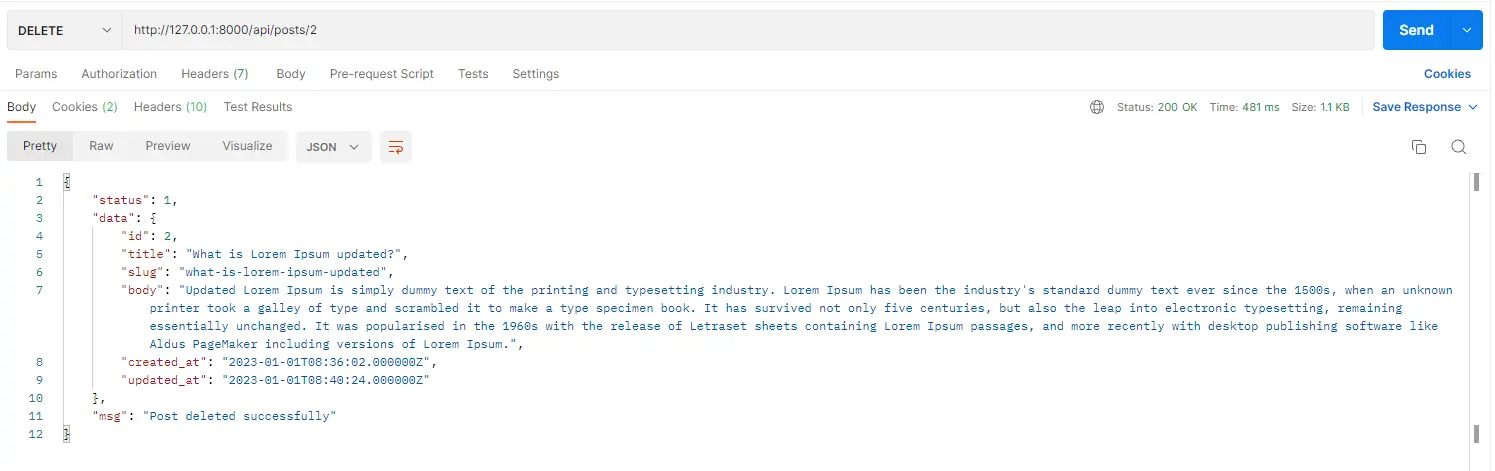
Concolusion
This way, you can create laravel rest apis for your mobile application. In this tutorial we have created a crud apis for our post crud operation. As you can see, we have not added any authentication to access these restful apis, but you can add user authentication to access these apis with laravel. Read this article to get detailed guidence on “How to authenticate rest apis with Laravel Passport?”
I hope it will be helpful, If you foud this tutorial helpful, share it will your fellows.
Happy Coding :)