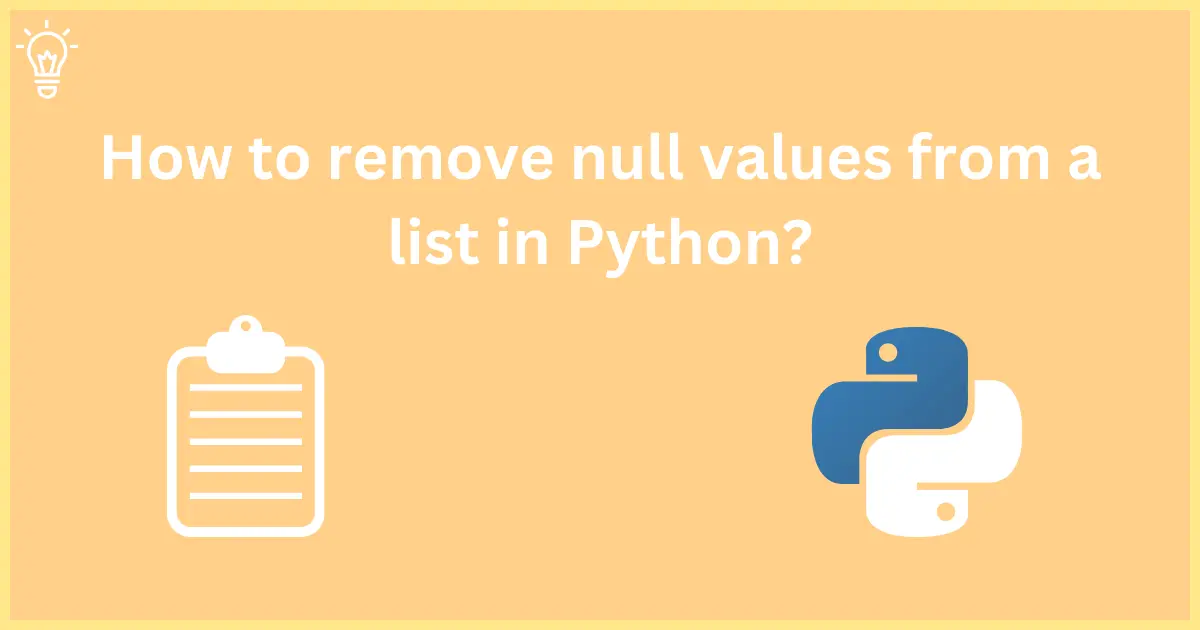
How to remove null values from a list in Python?
Hello Devs,
Today we are going to learn how to remove null values from a list in python. This article goes into details on how to remove empty values from the list in python. You will learn about various options to remove null values from a list with python. I will show you the few examples to remove none values from a list in python with different available methods.
So let's get started.
Method 1: Using the filter function
The filter()
function can be used to remove null values from the list. It will return a new iterator that excludes null values, then you can convert this iterator back to a list using the list function.
myList = ['', 'CodersVibe.com', '', 'is', 'Best', '', 'for', 'Coders']
# Remove NULL Values from the List
newList = list(filter(None, myList))
print(newList) # Output: ['CodersVibe.com', 'is', 'Best', 'for', 'Coders']
Method 2: Using the join function
You can use join()
method as an alternative to remove null values from the list in python.
myList = ['', 'CodersVibe.com', '', 'is', 'Best', '', 'for', 'Coders']
# Remove NULL Values from the List
newList = ' '.join(myList).split()
print(newList) # Output: ['CodersVibe.com', 'is', 'Best', 'for', 'Coders']
Method 3: Using the remove function
We can use remove function also with while loop to check each value if value is null.
myList = ['', 'CodersVibe.com', '', 'is', 'Best', '', 'for', 'Coders']
# Remove NULL Values from the List
while("" in myList) :
myList.remove("")
print(myList) # Output: ['CodersVibe.com', 'is', 'Best', 'for', 'Coders']
Method 4: Using a for loop
You can use for loop to remove null or none values from a list in python
original_list = ['', 'CodersVibe.com', '', 'is', 'Best', '', 'for', 'Coders']
new_list = []
for x in original_list:
if x != '':
new_list.append(x)
print(new_list) # Output: ['CodersVibe.com', 'is', 'Best', 'for', 'Coders']
Conclusion:
In conclusion, there are several ways to remove null values (i.e., null
values) from a list in Python. One common method is to use a remove()
or the filter()
function to create a new list that excludes null values. Another method is to use a for loop to iterate over the original list and append non-null values to a new list. Regardless of the method used, the end result is a new list that contains only non-null values from the original list. It's important to note that null values are not the same as empty strings (''
), so the condition used to remove null values should be appropriate for the data type being used.
I hope it will help you
Happy Coding :)