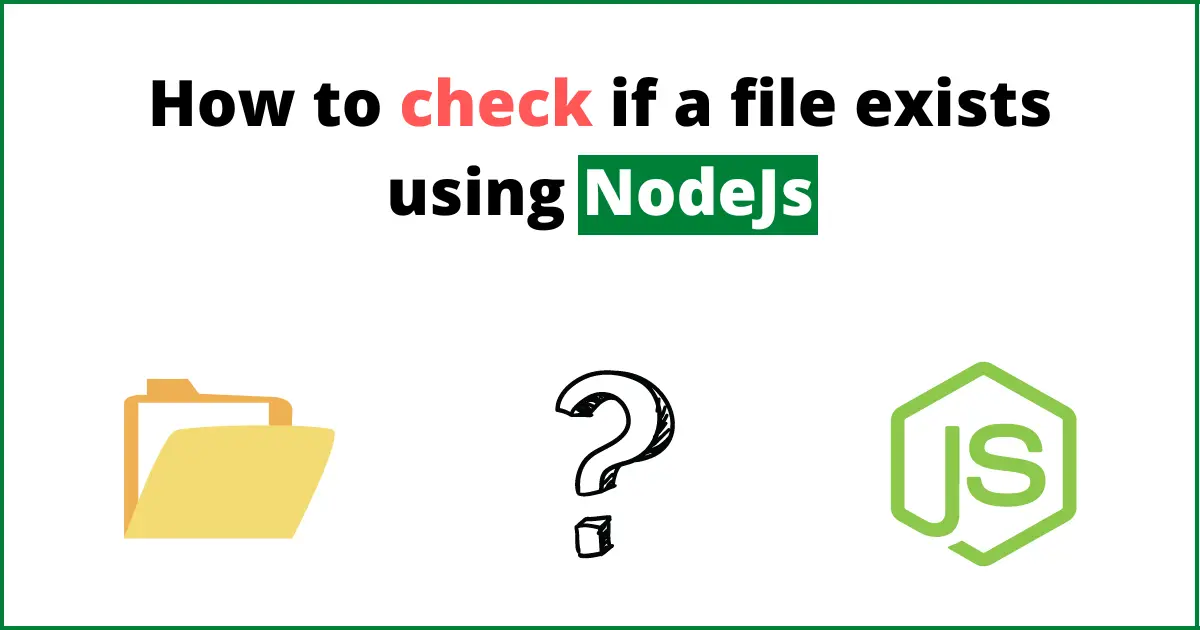
How to check if a file exists using NodeJs?
Hello Coders,
This tutorial goes into detail on how to check if a file or file path exists using NodeJS. I will show you two easy and quick examples to check if a file or a file path exists in the filesystem using NodeJs. There are two methods in NodeJs to check if a file exists or not in the directory.
So, let's get started.
Using fs.existsSync()
method
We can check a file's existence with the help of the fs.existsSync()
method. NodeJs provides a convenient and quick function to check if a file or a file path exists.
const fs = require('fs')
const path = './file.txt'
try {
if (fs.existsSync(path)) {
//file exists
}
} catch(err) {
console.error(err)
}
This method checks the file synchronously, which means it's blocking. But if you wanna check asynchronously, then you can use fs.access()
method. It works asynchronously, which means it checks the file without opening it.
Using fs.access()
method
const fs = require('fs')
const path = './file.txt'
fs.access(path, fs.F_OK, (err) => {
if (err) {
console.error(err)
return
}
//file exists
})
I hope, it will help you.
Happy Coding :)