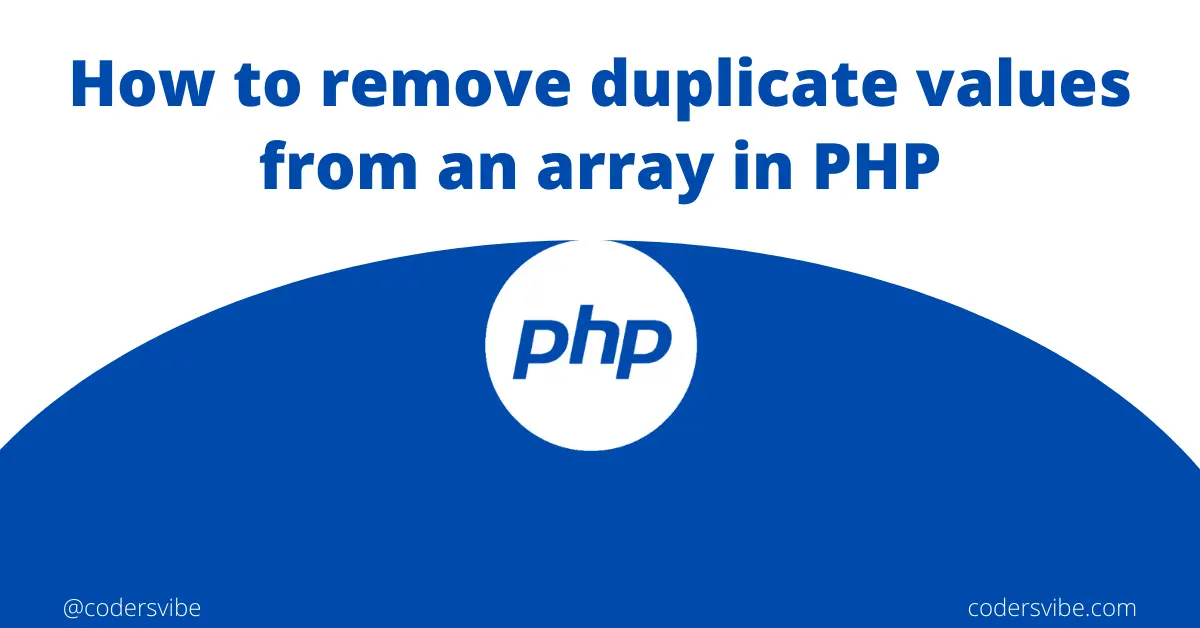
How to remove duplicate values from an array in PHP?
Hello Devs! In this post, I will show you How to remove duplicate values from an array in PHP. I will show you a simple example of removing duplicate values from an array. I will help you to give some examples of removing duplicate values from an array in PHP. The easy and simple answer is to use a PHP function array_unique()
. So, Let’s see below examples of removing duplicate values from an array in PHP.
Method 1: Using array_unique()
to remove Duplicate values
In this method, we will use array_unique()
the function of PHP to remove duplicate values from an array, we will pass the indexed array as its argument.
Removing from an Indexed Array in PHP
<?php
//Declare an indexed array in PHP
$myarrayind = array("Cycle", "Bike", "Car", "Cycle", "Bolero", "Car");
//Remove duplicate indexed array elements using array_unique()
$RemDupArrInd = array_unique($myarrayind);
//Print result
print_r($RemDupArrInd);
?>
Output:
Array
(
[0] => Cycle
[1] => Bike
[2] => Car
[4] => Bolero
)
The given above example is contains the indexed array variable with duplicate values. After the use of array_unique()
the function, the output gives the new indexed array with unique values.
Removing from an Associative Array in PHP
Similarly, we can remove the duplicate values from an associative array using array_unique()
function. The function takes a single argument as an associative array. Let's see the example to get the exact point of what I wanna show you.
<?php
//Declare an associative array in PHP
$myarrayassoc = array("Cycle" => 2, "Bike" => 5, "Cycle" => 2, "Car" => 9, "Bike" => 5);
//Remove duplicate associative array elements using array_unique()
$RemDupArrAssoc = array_unique($myarrayassoc);
//Print result
print_r($RemDupArrAssoc);
?>
Output:
Array
(
[Cycle] => 2
[Bike] => 5
[Car] => 9
)
The output will give you a new associative array with unique values.
Read also: Get all keys from an array that starts with a certain string
Method 2: Using Foreach Loop to remove Duplicate Values From an Array
The Foreach loop of PHP can also be used to remove the duplicate values from an array in PHP. So, let's do it with separate examples for indexed and associative arrays.
Removing from Indexed Array in PHP
To use the foreach loop to remove the duplicate values from an indexed array in PHP, first, we will declare an empty array. After that, use the foreach loop inside, we will check the array in if condition using the in_array()
function.
Further, the unique values get added to the empty array on each iteration. Let's see the example given below to learn more clearly.
<?php
//Declare an indexed array in PHP
$myarrayind = array("Cycle", "Bike", "Car", "Cycle", "Bolero", "Car");
//Deleting duplicate indexed array elements using Foreach loop
$RemDupArrInd = array();
$i=0;
foreach ($myarrayind as $indvalue){
if(!in_array($indvalue, $RemDupArrInd))
$RemDupArrInd[$i]=$indvalue;
$i++;
}
//Print result
print_r($RemDupArrInd);
?>
Output:
Array
(
[0] => Cycle
[1] => Bike
[2] => Car
[4] => Bolero
)
Deleting from an Associative Array in PHP
Similarly, to remove the duplicate values from an associative array in PHP, we can use the foreach loop. It also requires declaring an empty array first. After that, we will use the foreach loop with the if condition to check the array using the in_array()
function inside it.
Likewise, on each iteration of the foreach loop, the unique values will be added to the array.
<?php
//Declare an associative array in PHP
$myarrayassoc = array("Cycle" => 2, "Bike" => 5, "Cycle" => 2, "Car" => 9, "Bike" => 5);
//Deleting duplicate associative array elements using Foreach loop
$RemDupArrAssoc = array();
foreach ($myarrayassoc as $assockey => $assocvalue){
if(!in_array($assocvalue, $RemDupArrAssoc))
$RemDupArrAssoc[$assockey]=$assocvalue;
}
//Print result
print_r($RemDupArrAssoc);
?>
Output:
Array
(
[Cycle] => 2
[Bike] => 5
[Car] => 9
)
The above-given example shows the output that contains the unique associative array values.
You can use any of the methods given above to remove duplicate values from an indexed and associative array in PHP. However, the array_unique()
function gives a faster result than the foreach loop.
How to remove duplicate values from Multidimensional Array in PHP
In addition to the above examples, we can also use the array_unique()
function and pass two arguments to it. The first one will be a multidimensional array and the second one will be the SORT_REGULAR
keyword.
We will get a multidimensional array with unique values as a result.
<?php
//Declare an indexed array in PHP
$mymultiarray = array(
array("Cycle" => 2, "Bike" => 5, "Car" => 9),
array("Cycle" => 3, "Bike" => 6, "Car" => 10),
array("Cycle" => 5, "Bike" => 8, "Car" => 12),
array("Cycle" => 3, "Bike" => 6, "Car" => 10),
array("Cycle" => 2, "Bike" => 5, "Car" => 9),
array("Cycle" => 3, "Bike" => 6, "Car" => 10),
array("Cycle" => 5, "Bike" => 8, "Car" => 12),
array("Cycle" => 3, "Bike" => 6, "Car" => 10),
array("Cycle" => 2, "Bike" => 5, "Car" => 9)
);
//Remove duplicate multidimensional array elements using array_unique()
$RemDupArrMulti = array_unique($mymultiarray, SORT_REGULAR);
//Print result
print_r($RemDupArrMulti);
?>
Output:
Array
(
[0] => Array ( [Cycle] => 2 [Bike] => 5 [Car] => 9 )
[1] => Array ( [Cycle] => 3 [Bike] => 6 [Car] => 10 )
[2] => Array ( [Cycle] => 5 [Bike] => 8 [Car] => 12 )
)
So, the result will show the multidimensional array with unique values.
To sum up, we can use array_unique()
it to get the unique elements from an array in PHP. However, for the multidimensional array, we have to use the SORT_REGULAR
keyword as the second parameter of array_unique()
a function.
I hope it will help you.
Happy Coding :)