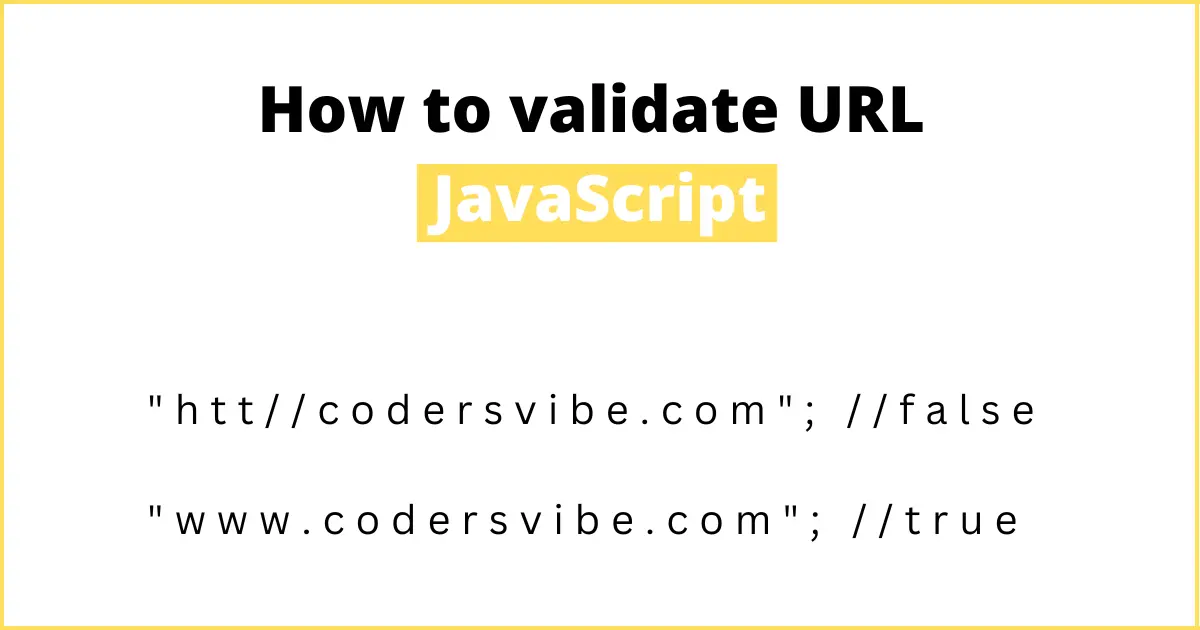
How to validate URL? - JavaScript
Hello Coders.
Today, We will discuss about how to validate URL in JavaScript. I will show you quick examples to check if a string is a valid URL. You will learn about to validate URL in JavaScript with RegExp.
So, let's get started to validate.
Create a function to validate URL with Regex
We will create a function to validate URLs. It is good approach to create functions to prevent code duplication and keeping your code clean and concise.
<script>
function checkURL(URL) {
var pattern = new RegExp(`^(https?:\\/\\/)?((([a-z\\d]([a-z\\d-]*[a-z\\d])*)\\.)+[a-z]{2,}|((\\d{1,3}\\.){3}\\d{1,3}))(\\:\\d+)?(\\/[-a-z\\d%_.~+]*)*(\\?[;&a-z\\d%_.~+=-]*)?(\\#[-a-z\\d_]*)?$`, 'i');
return !!pattern.test(URL);
}
</script>
We have created a function, now we can use it anywhere in our JavaScript file to validate URL.
Read also: How to validate email address in ReactJs?
<script>
var url = "invalidURL";
console.log(checkURL(url)); //false
var url = "htt//codersvibe.com"; //false
console.log(checkURL(url));
var url = "www.codersvibe.com"; //true
console.log(checkURL(url));
var url = "https://www.codersvibe.com"; //true
console.log(checkURL(url));
var url = "https://www.codersvibe.com/how-to-remove-last-item-from-array-in-javascript";
console.log(checkURL(url)); //true
</script>
Conclusion
In this tutorial, we have learned how to validate URL in JavaScript. We have created a simple function to validate URL with Regex Expressions.
I hope, it will help you.
Happy Learning :)