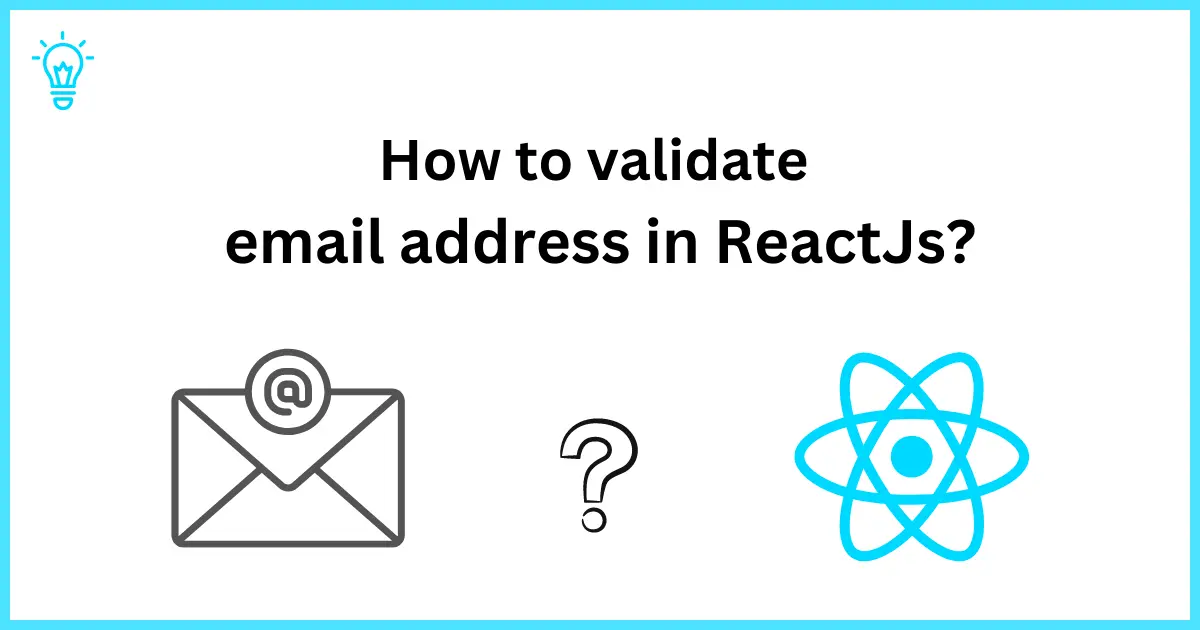
How to validate email address in ReactJs?
Hello Guys,
Email validation is a key part of any application. Because it is compulsory to prevent users from making typical mistakes at the time of registration or restrict using a valid email because it will be used for many purposes after registration, like login, sending emails and other means of communication. In this tutorial we will learn about how to validate an email address in a ReactJs application. Whatever method you use for email validation in ReactJs, you can not do it without regular expressions. It is also called regex validation. Regex is a pattern that is used to match the combination of characters in a string. Mind that in JavaScript, regex is also an object.
So let's get started.
Method 1: With Formik Library
Formik is a react library to create and validate the form inputs “without the tears”. First, we need to install the npm package in our react application.
npm i formik
Then add the useFormik hook to your form component.
import { useFormik } from 'formik';
Now add the following useFormik hook structure:
const formik = useFormik({
initialValues: {
email: '',
},
validate,
onSubmit: (values) => {
alert(JSON.stringify(values, null, 2))
},
})
Where initialValues is an object of default values and the onSubmit function is called when the form is submitted and the email validation is passed. The validate function is a separate function that is responsible for handling our validation process. Here's what our validation function will look like:
const validate = (values) => {
const errors = {}
if (!values.email) {
errors.email = 'Required'
} else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i.test(values.email)) {
errors.email = 'Invalid email address'
}
return errors
}
Let's bind the code in one place. Here's what our complete email validation component will look like:
import React from 'react';
import { useFormik } from 'formik'
const validate = (values) => {
const errors = {}
if (!values.email) {
errors.email = 'Required'
} else if (!/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i.test(values.email)) {
errors.email = 'Invalid email address'
}
return errors
}
export default function EmailForm() {
const formik = useFormik({
initialValues: {
email: '',
},
validate,
onSubmit: (values) => {
alert(JSON.stringify(values, null, 2))
},
})
return (
<div>
<form onSubmit={formik.handleSubmit}>
<div>
<label for="email">Email</label>
<input type="email" name="email" id="email"
onChange={formik.handleChange} onBlur={formik.handleBlur} value={formik.values.email} />
{formik.touched.email && formik.errors.email && (
<span>{formik.errors.email}</span>
)}
<button type='submit'>Submit</button>
</div>
</form>
</div>
);
}
Read also: How to validate URL in JavaScript?
Method 2: With React Hook Form library
React Hook Form is the famous library to validate the forms in ReactJs because of its easy to use syntax and short lines of code. Now let's see how we can use it. First we need to install the library.
npm install react-hook-form
Now, let's get to an understanding of the basic functions that will be used in the validation.
useForm
First we will use a useForm hook that contains all the required parameters that will be used in the validation process.
register()
This function registers all the required fields and all validation options can, so the useForm hook can use these options and run the validation process.
Here is the complete code of the form component with React Hook From Library.
import React from 'react';
import { useForm } from 'react-hook-form';
export default function EmailForm() {
const {
register,
handleSubmit,
formState: { errors }
} = useForm();
const onSubmit = (values) => alert(JSON.stringify(values, null, 2));
return (
<form onSubmit={handleSubmit(onSubmit)}>
<input
type="text"
placeholder="Email"
{...register("email", { required: true, pattern: /^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i })}
/>
{errors.email && <span>Invalid email</span>}
<input type="submit" />
</form>
);
}
Hint: Here you can generate your form code with controls.
Method 3: With the validator module
The alternative way of email validation is to use the Validator Module in react. It is a pretty straightforward and easy to use method.
Let's install the validator npm package first.
npm install validator
Our main focus is the email validation functionality, so we will not get into UI details. It will be as simple as it can be. Let's update your EmailForm component with the following lines of code.
import React, { useState } from "react";
import { render } from "react-dom";
import validator from "validator";
export default function EmailForm() {
const [message, setMessage] = useState("");
const validateEmail = (e) => {
var email = e.target.value;
if (validator.isEmail(email)) {
setMessage("Thank you");
} else {
setMessage("Please, enter valid Email!");
}
};
return (
<div>
<h2>Validating Email in ReactJS</h2>
<span>Enter Email: </span>
<input
type="text"
id="userEmail"
onChange={(e) => validateEmail(e)}
></input>
<br />
<span
style={{
fontWeight: "bold",
color: "red"
}}
>
{message}
</span>
</div>
);
}
You can check the form by running the start command:
npm run start
And you can make it fancy as per your needs.
Method 4: Let's write our own few lines of code
There might be a thousand ways to validate an email but the one question comes: can we do it manually with our own code? Yes, we can do it manually without any libraries. So, let's go for the one that is simple and written to speed up the process of validation.
Update your EmailForm component with the following code:
import React, { useState } from "react";
import { render } from "react-dom";
const isEmail = (email) =>
/^[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,4}$/i.test(email);
export default function EmailForm() {
const [values, setValues] = useState({ email: "" });
const [errors, setErrors] = useState({});
const validateAndSubmitForm = (e) => {
e.preventDefault();
const errors = {};
if (!isEmail(values.email)) {
errors.email = "Wrong email";
}
setErrors(errors);
if (!Object.keys(errors).length) {
alert(JSON.stringify(values, null, 2));
}
};
const setEmail = (e) => {
setValues((values) => ({ ...values, email: e.target.value }));
};
return (
<form onSubmit={validateAndSubmitForm}>
<h2>Validating Email in ReactJS</h2>
<span>Enter Email: </span>
<input
type="text"
id="userEmail"
value={values.email}
onChange={setEmail}
/>{" "}
<input type="submit" />
<br />
{Object.entries(errors).map(([key, error]) => (
<span
key={`${key}: ${error}`}
style={{
fontWeight: "bold",
color: "red"
}}
>
{key}: {error}
</span>
))}
</form>
);
}
Conclusion:
I have highlighted the various methods for email validation in ReactJs. In this detailed tutorial, i have covered the Formik library, React Hook Form, as well as leveraging the validator npm package. Additionally, I have provided a custom method to validate the email using regular expressions. Every method ensures proper email validation for your ReactJs applications.
I hope it will help you.
Happy Coding :)