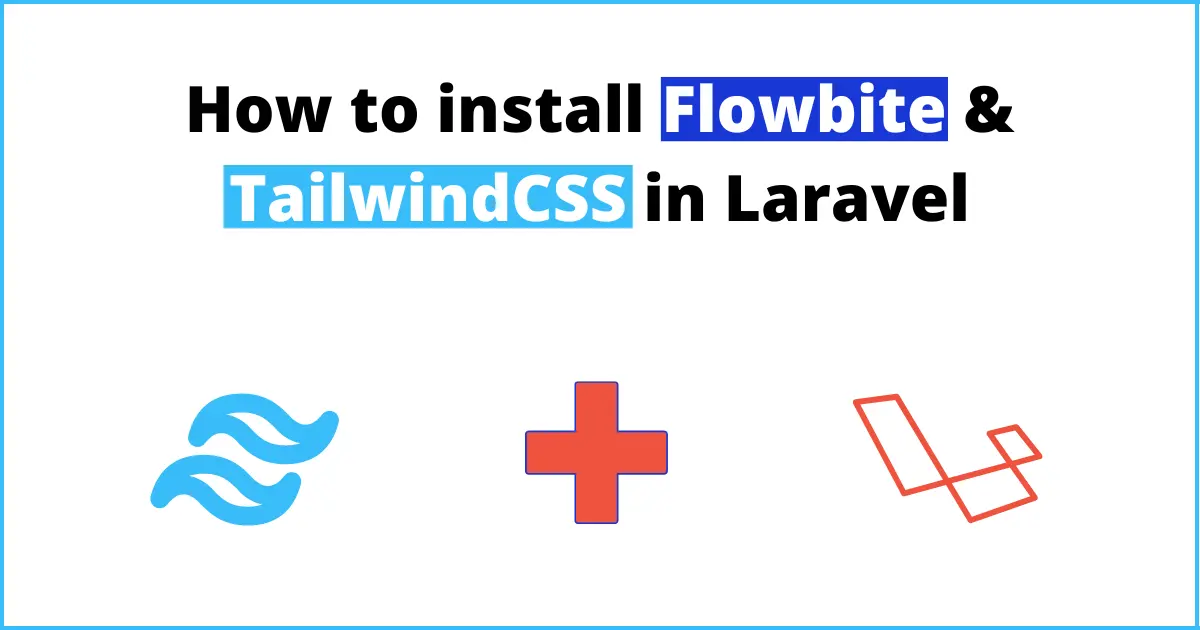
How to install Flowbite & TailwindCSS in Laravel?
Hello Devs,
In this tutorial we will install and setup flowbite in laravel application. We will add flowbite with laravel breeze. Flowbite can be added as a plugin with npm package installer into an existing tailwindCSS project. In our case we will install a new laravel 8 project with breeze starter kit and then we will add flowbite as a plugin with npm package installer.
You can skip first 2 steps if you have already a laravel project installed.
Step 1: Install Laravel
In this article, i will use laravel 8, you can use laravel 9 also. To install a 8th version of laravel, you need specify the version like this following command.
composer create-project laravel/laravel:^8.0 example-app
But, if you wanna use laravel 9, you can simply run this command without any version specification.
composer create-project laravel/laravel example-app
Note: You can change example-app
to your own name.
Step 2: Install Breeze for laravel Starter Kit
We will use laravel breeze package for creating a starter kit. In starter kit we will get a tailwindCSS scaffolding with all authentication routes to just start our project. To install laravel breeze run this command:
composer require laravel/breeze:1.9.2
But if you using laravel 9 then you don't need to specify the breeze version, you can just run this command:
composer require laravel/breeze --dev
We need to run this commands to publish config file.
php artisan breeze:install
Install and run npm
npm install
npm run dev
Now serve your laravel app.
php artisan serve
Step 3: Install & setup Flowbite in laravel
We will install flowbite via npm packager installer.
npm install flowbite
Now, need to add flowbite as a plugin in the tailwind.config.js file.
/tailwind.config.js
const defaultTheme = require('tailwindcss/defaultTheme');
module.exports = {
content: [
'./vendor/laravel/framework/src/Illuminate/Pagination/resources/views/*.blade.php',
'./storage/framework/views/*.php',
'./resources/views/**/*.blade.php',
],
theme: {
extend: {
fontFamily: {
sans: ['Nunito', ...defaultTheme.fontFamily.sans],
},
},
},
plugins: [require('@tailwindcss/forms'), require('flowbite/plugin')],
};
It is require to add flowbite.js
file before the end of the <body/>
tag:
<script src="{{ asset('../path/to/flowbite/dist/flowbite.js') }}"></script>
Or we can also add this using CDN:
<script src="https://unpkg.com/[email protected]/dist/flowbite.js"></script>
views/layouts/guest.blade.php
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<meta name="csrf-token" content="{{ csrf_token() }}">
<title>{{ config('app.name', 'Laravel') }}</title>
<!-- Fonts -->
<link rel="stylesheet" href="https://fonts.googleapis.com/css2?family=Nunito:wght@400;600;700&display=swap">
<!-- Styles -->
<link rel="stylesheet" href="{{ asset('css/app.css') }}">
<!-- Scripts -->
<script src="{{ asset('js/app.js') }}" defer></script>
</head>
<body>
<div class="font-sans text-gray-900 antialiased">
{{ $slot }}
</div>
<script src="https://unpkg.com/[email protected]/dist/flowbite.js"></script>
</body>
</html>
Test flowbite configuration with any tailwind component in laravel blade file. I will use Tailwind CSS Accordion
views/test.blade.php
<x-guest-layout>
<div id="accordion-collapse" data-accordion="collapse">
<h2 id="accordion-collapse-heading-1">
<button type="button"
class="flex items-center justify-between w-full p-5 font-medium text-left border border-b-0 border-gray-200 rounded-t-xl focus:ring-4 focus:ring-gray-200 dark:focus:ring-gray-800 dark:border-gray-700 hover:bg-gray-100 dark:hover:bg-gray-800 bg-gray-100 dark:bg-gray-800 text-gray-900 dark:text-white"
data-accordion-target="#accordion-collapse-body-1" aria-expanded="true"
aria-controls="accordion-collapse-body-1">
<span>What is Flowbite?</span>
<svg data-accordion-icon="" class="w-6 h-6 rotate-180 shrink-0" fill="currentColor" viewBox="0 0 20 20"
xmlns="http://www.w3.org/2000/svg">
<path fill-rule="evenodd"
d="M5.293 7.293a1 1 0 011.414 0L10 10.586l3.293-3.293a1 1 0 111.414 1.414l-4 4a1 1 0 01-1.414 0l-4-4a1 1 0 010-1.414z"
clip-rule="evenodd"></path>
</svg>
</button>
</h2>
<div id="accordion-collapse-body-1" class="" aria-labelledby="accordion-collapse-heading-1">
<div class="p-5 font-light border border-b-0 border-gray-200 dark:border-gray-700 dark:bg-gray-900">
<p class="mb-2 text-gray-500 dark:text-gray-400">Flowbite is an open-source library of interactive
components built on top of Tailwind CSS including buttons, dropdowns, modals, navbars, and more.</p>
<p class="text-gray-500 dark:text-gray-400">Check out this guide to learn how to <a
href="/docs/getting-started/introduction/"
class="text-blue-600 dark:text-blue-500 hover:underline">get started</a> and start developing
websites even faster with components on top of Tailwind CSS.</p>
</div>
</div>
<h2 id="accordion-collapse-heading-2">
<button type="button"
class="flex items-center justify-between w-full p-5 font-medium text-left text-gray-500 border border-b-0 border-gray-200 focus:ring-4 focus:ring-gray-200 dark:focus:ring-gray-800 dark:border-gray-700 dark:text-gray-400 hover:bg-gray-100 dark:hover:bg-gray-800"
data-accordion-target="#accordion-collapse-body-2" aria-expanded="false"
aria-controls="accordion-collapse-body-2">
<span>Is there a Figma file available?</span>
<svg data-accordion-icon="" class="w-6 h-6 shrink-0" fill="currentColor" viewBox="0 0 20 20"
xmlns="http://www.w3.org/2000/svg">
<path fill-rule="evenodd"
d="M5.293 7.293a1 1 0 011.414 0L10 10.586l3.293-3.293a1 1 0 111.414 1.414l-4 4a1 1 0 01-1.414 0l-4-4a1 1 0 010-1.414z"
clip-rule="evenodd"></path>
</svg>
</button>
</h2>
<div id="accordion-collapse-body-2" class="hidden" aria-labelledby="accordion-collapse-heading-2">
<div class="p-5 font-light border border-b-0 border-gray-200 dark:border-gray-700">
<p class="mb-2 text-gray-500 dark:text-gray-400">Flowbite is first conceptualized and designed using the
Figma software so everything you see in the library has a design equivalent in our Figma file.</p>
<p class="text-gray-500 dark:text-gray-400">Check out the <a href="https://flowbite.com/figma/"
class="text-blue-600 dark:text-blue-500 hover:underline">Figma design system</a> based on the
utility classes from Tailwind CSS and components from Flowbite.</p>
</div>
</div>
<h2 id="accordion-collapse-heading-3">
<button type="button"
class="flex items-center justify-between w-full p-5 font-medium text-left text-gray-500 border border-gray-200 focus:ring-4 focus:ring-gray-200 dark:focus:ring-gray-800 dark:border-gray-700 dark:text-gray-400 hover:bg-gray-100 dark:hover:bg-gray-800"
data-accordion-target="#accordion-collapse-body-3" aria-expanded="false"
aria-controls="accordion-collapse-body-3">
<span>What are the differences between Flowbite and Tailwind UI?</span>
<svg data-accordion-icon="" class="w-6 h-6 shrink-0" fill="currentColor" viewBox="0 0 20 20"
xmlns="http://www.w3.org/2000/svg">
<path fill-rule="evenodd"
d="M5.293 7.293a1 1 0 011.414 0L10 10.586l3.293-3.293a1 1 0 111.414 1.414l-4 4a1 1 0 01-1.414 0l-4-4a1 1 0 010-1.414z"
clip-rule="evenodd"></path>
</svg>
</button>
</h2>
<div id="accordion-collapse-body-3" class="hidden" aria-labelledby="accordion-collapse-heading-3">
<div class="p-5 font-light border border-t-0 border-gray-200 dark:border-gray-700">
<p class="mb-2 text-gray-500 dark:text-gray-400">The main difference is that the core components from
Flowbite are open source under the MIT license, whereas Tailwind UI is a paid product. Another
difference is that Flowbite relies on smaller and standalone components, whereas Tailwind UI offers
sections of pages.</p>
<p class="mb-2 text-gray-500 dark:text-gray-400">However, we actually recommend using both Flowbite,
Flowbite Pro, and even Tailwind UI as there is no technical reason stopping you from using the best
of two worlds.</p>
<p class="mb-2 text-gray-500 dark:text-gray-400">Learn more about these technologies:</p>
<ul class="pl-5 text-gray-500 list-disc dark:text-gray-400">
<li><a href="https://flowbite.com/pro/"
class="text-blue-600 dark:text-blue-500 hover:underline">Flowbite Pro</a></li>
<li><a href="https://tailwindui.com/" rel="nofollow"
class="text-blue-600 dark:text-blue-500 hover:underline">Tailwind UI</a></li>
</ul>
</div>
</div>
</div>
</x-guest-layout>
Run npm
npm run dev
You can run this command also for continuously watching updates in config files.
npm run watch
Result:

You can find complete source code here.
I hope, it will help you.
Happy Coding :)