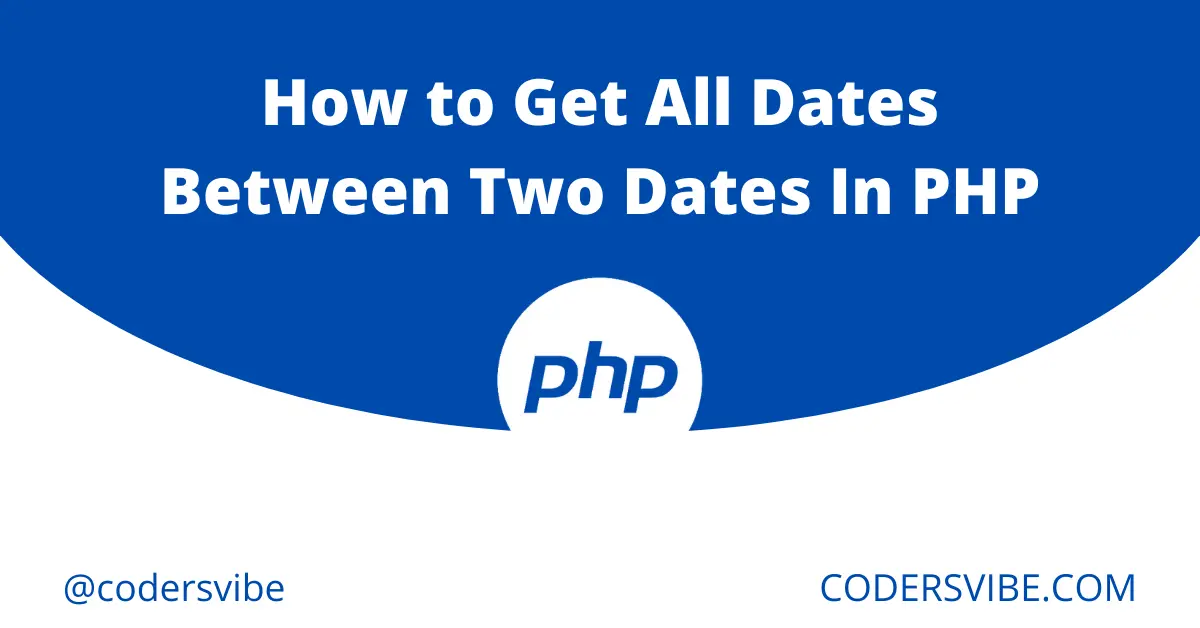
How to get all dates between two dates in PHP?
Hello Developers! In this post, I will show you, How to get all dates between two dates in PHP. This article goes into detail on how to get all dates between two dates in PHP with a few lines of code. I will give you Two simple and easy examples with solutions. So let's start to get dates between two dates.
Example 1: Get all dates between two dates with strtotime function
In the first example, we will use strtotime()
function to get all dates between two dates.
Note:
- The
strtotime()
function is a built-in function in PHP that is used to convert an English textual date-time description to a UNIX timestamp. - There are 86,400 seconds in 1 day, So, I have use 86400 in for loop.
<?php
function getAllDates($startingDate, $endingDate)
{
$datesArray = [];
$startingDate = strtotime($startingDate);
$endingDate = strtotime($endingDate);
for ($currentDate = $startingDate; $currentDate <= $endingDate; $currentDate += (86400)) {
$date = date('Y-m-d', $currentDate);
$datesArray[] = $date;
}
return $datesArray;
}
$dates = getAllDates('2021-01-01', '2021-01-10');
print_r($dates);
?>
Output:
Array (
[0] => 2021-01-01
[1] => 2021-01-02
[2] => 2021-01-03
[3] => 2021-01-04
[4] => 2021-01-05
[5] => 2021-01-06
[6] => 2021-01-07
[7] => 2021-01-08
[8] => 2021-01-09
[9] => 2021-01-10
)
Example 2: Get all dates with DateInterval function
In the second example, We will use another PHP function called by DateInterval()
.
Note:
- A date interval stores either a fixed amount of time (in years, months, days, hours etc) or a relative time string in the format that DateTime's constructor supports. More specifically, the information in an object of the DateInterval class is an instruction to get from one date/time to another date/time. you can visit php.net for more information about this function.
- This function will not show last date e.g If we wanna get dates from 1 to 10, It will show all dates from 1 to 9 not will show the last date.
<?php
$datesArray = [];
$timePeriod = new DatePeriod(
new DateTime('2021-01-01'),
new DateInterval('P1D'),
new DateTime('2021-01-10')
);
foreach ($timePeriod as $key => $value) {
$date = $value->format('Y-m-d');
$datesArray[] = $date;
}
print_r($datesArray);
?>
Output:
Array (
[0] => 2021-01-01
[1] => 2021-01-02
[2] => 2021-01-03
[3] => 2021-01-04
[4] => 2021-01-05
[5] => 2021-01-06
[6] => 2021-01-07
[7] => 2021-01-08
[8] => 2021-01-09
)
I hope it can be helpful for you.
Happy Coding :)