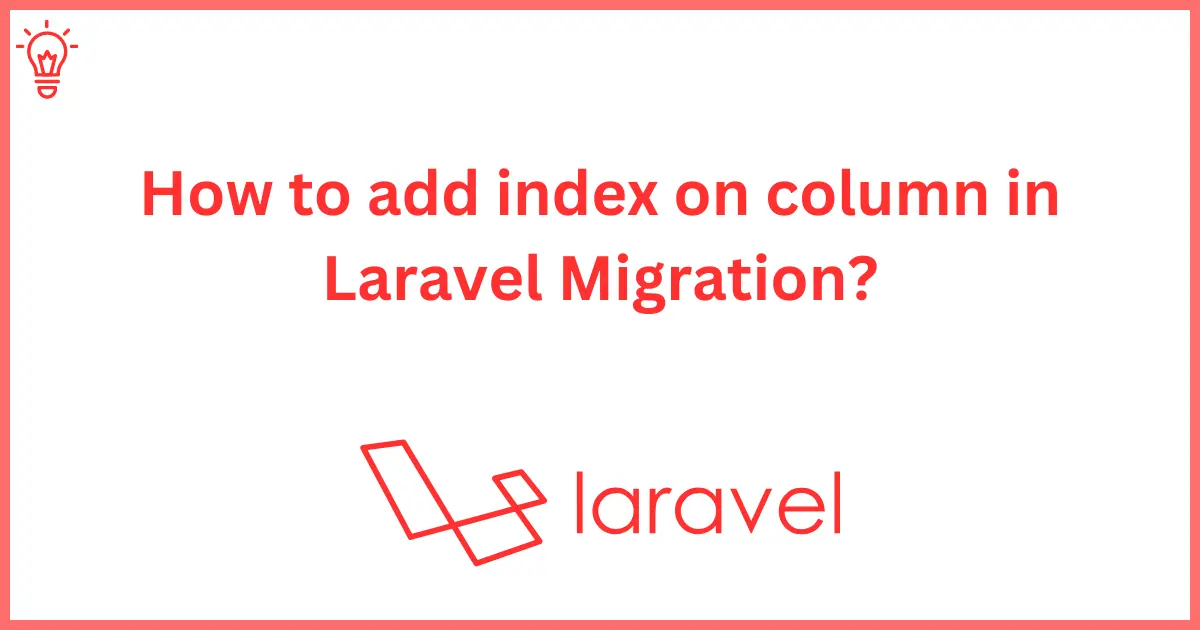
How to add index on column in Laravel Migration?
In this tutorial, you will learn how to add index on column in laravel migration. If you are going to create search functionality and want a column to be searched, then it is good practice to add an index to that column. This tutorial goes into detail on how to add the mysql index to the laravel migration file. This quick tutorial will show you how to add a unique mysql index to any column in laravel migration.
So let's get started.
Add index on a single column
In this example, we will see how to add a mysql index to a single column in a laravel migration file. We will create a testing table named test1 to add an index to the column.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('test1', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('body')->index();
$table->boolean('status')->default(false);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('posts');
}
};
The above example will add an index to the body column as you can see in the following preview.
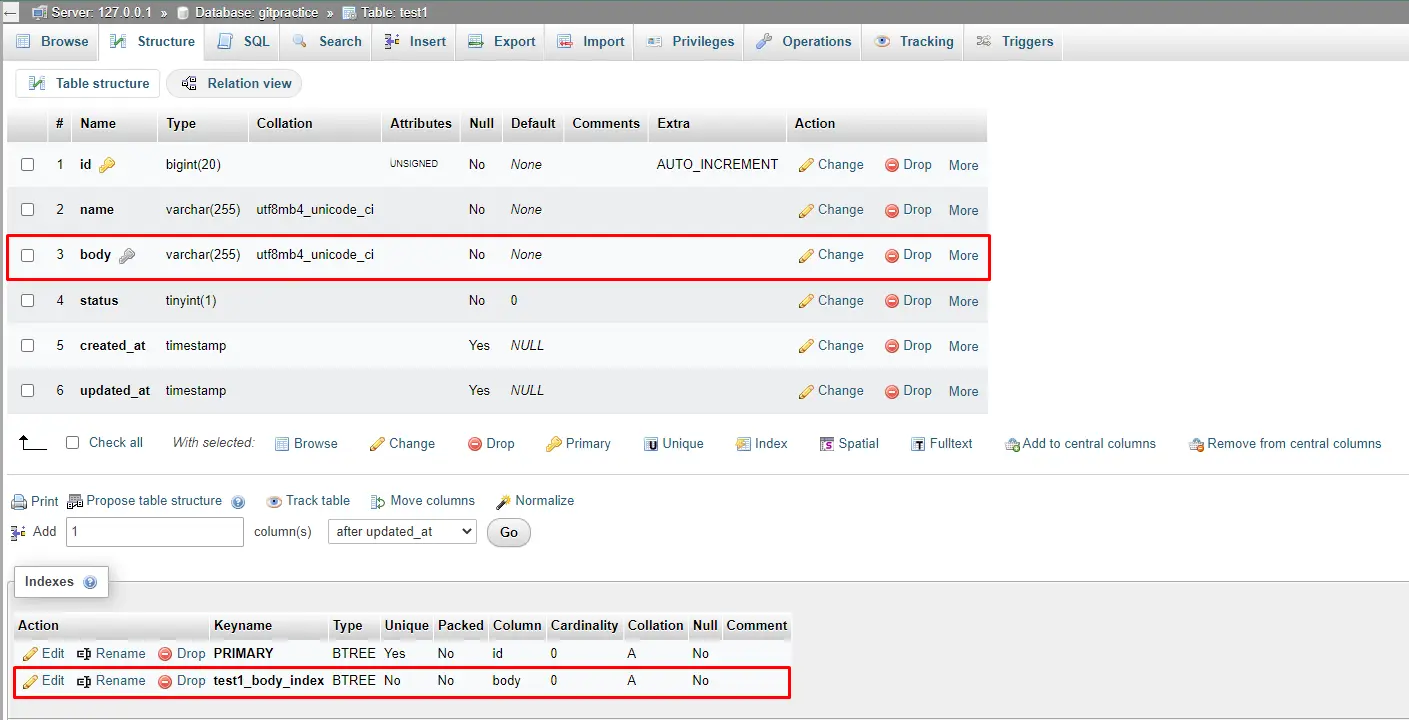
Add index on multiple columns
You can add index on multiple columns in larave migration with one command and at a same time. We will create a testing table named test2
to add index on column. Let's see the following example:
<?php
use App\Models\User;
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('test2', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('body');
$table->boolean('status')->default(false);
$table->index(['name', 'body']);
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::dropIfExists('test2');
}
};
The above example of code will add index on name and body column. Check following preview.
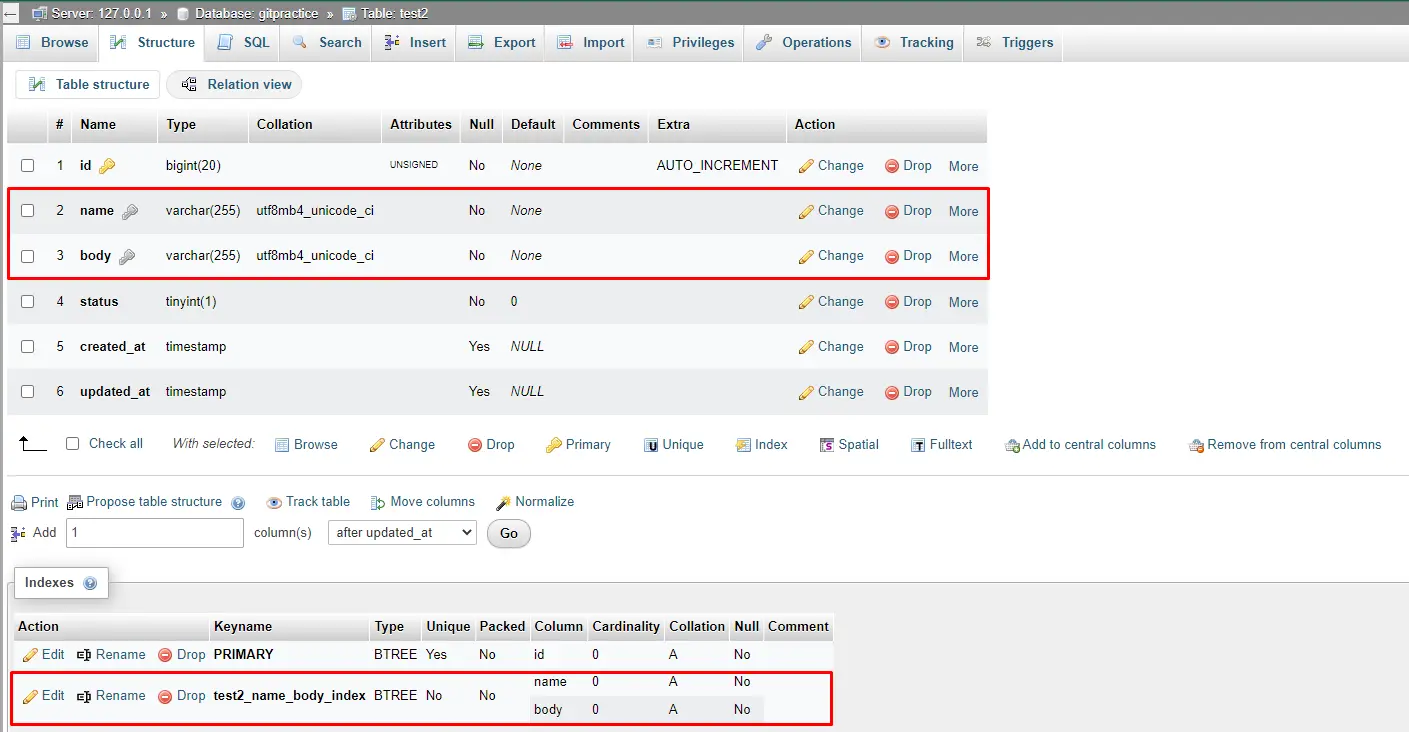
Run php artisan migrate
command to migrate the table to the database.
Conclusion:
In this short tutorial, i have tried to explain you, how to add index on single columns and multiple columns in laravel migration. I hope this tutorial will help you to add mysql index on column in laravel migration.
Happy Coding :)