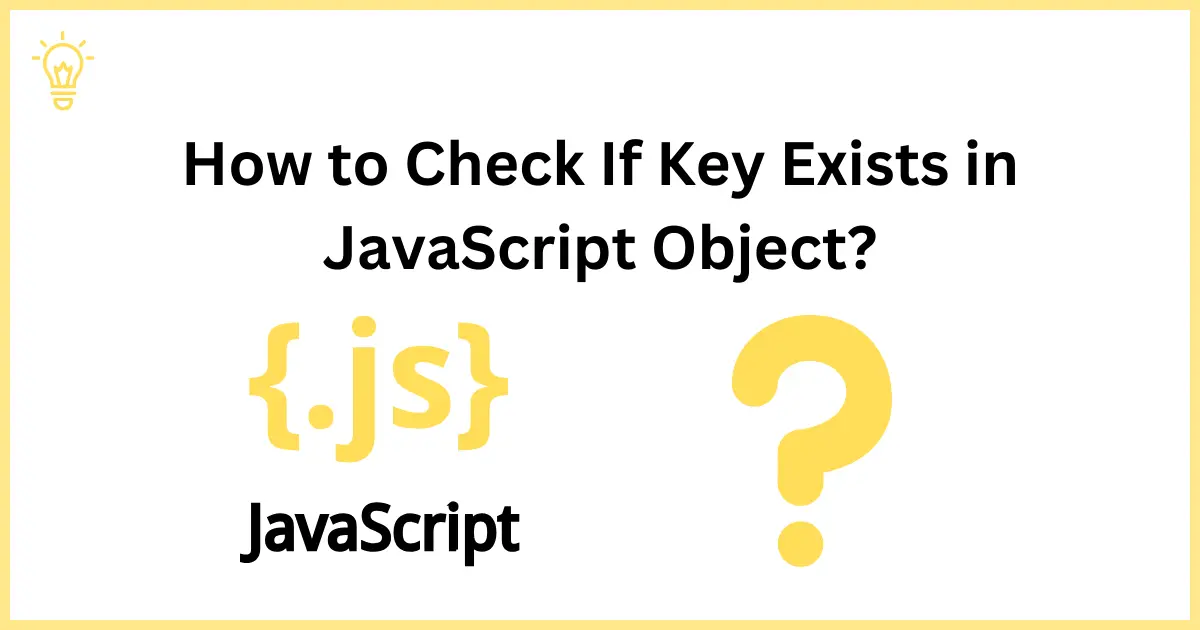
How to Check If Key Exists in JavaScript Object?
Do you often find yourself wondering how to check if a key exists in object? This detailed article will resolve the mystery. Discover the methods that will simplified your code and make your development journey smoother.
There are many methods to check the existence of a key in the object. Let's explore following methods one by one.
1: Using the 'in' Operator:
Easily check if a key exists in object with the 'in' operator. It's as simple as key
'in
' object
. This clear-cut approach lets you quickly determine key existence.
const myObject = { name: 'John', age: 25 };
if ('name' in myObject) {
// Key 'name' exists!
}
2: Using hasOwnProperty:
Dig deeper with hasOwnProperty
. This method ensures that the key is a direct property of the object, not inherited from its prototype.
const myObject = { name: 'John', age: 25 };
if (myObject.hasOwnProperty('name')) {
// Key 'name' is a direct property!
}
3: Using Undefined Check
Employ a simple undefined check to verify key existence. This method works best when you want to ensure the key has a value.
const myObject = { name: 'John', age: 25 };
if (myObject['name'] !== undefined) {
// Key 'name' has a value!
}
Conclusion
Today, we explored essential techniques for checking the existence of keys in JavaScript objects. Exploring three distinct methods – the 'in'
operator, hasOwnProperty
, and an undefined check – provide you with versatile tools to streamline your code. Implementing these approaches will undoubtedly enhance your coding proficiency, providing a solid foundation for navigating and optimizing your JavaScript projects.
I hope it will help you.
Happy coding!