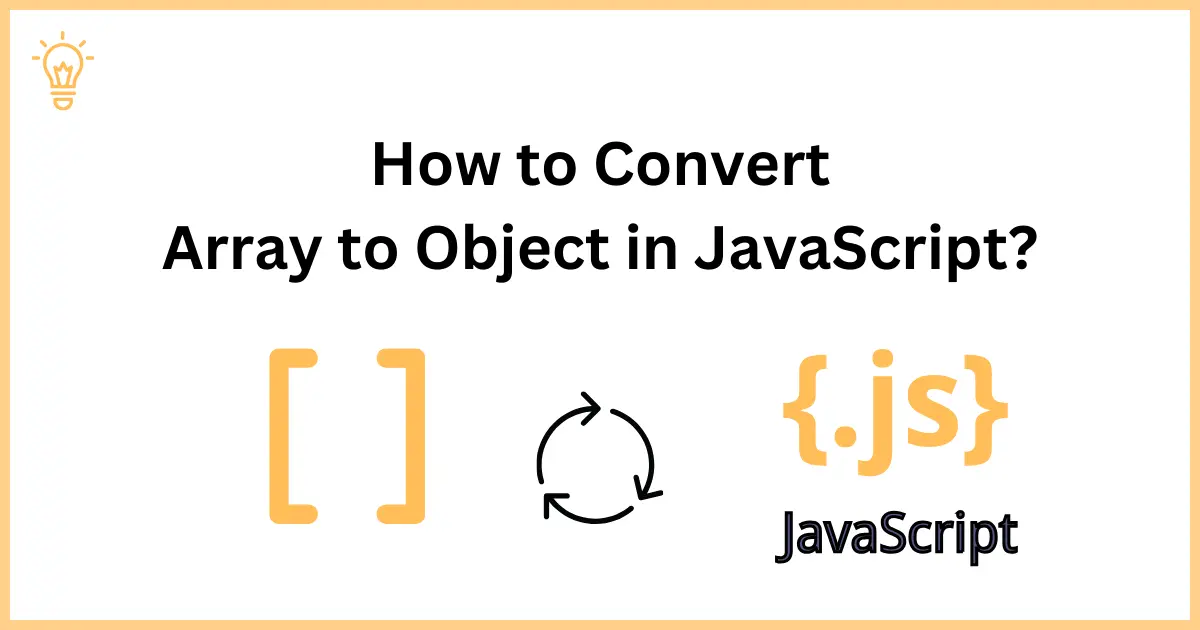
How to Convert Array to Object in JavaScript?
Have you ever needed to convert an array into a json object? Today, we are going to learn about how to convert array to object in JavaScript. We will use the Object.assign()
function to convert an array into a JSON object in JavaScript. There will be a quick tutorial regarding converting arrays into objects with a few lines of code.
So let's see the following examples.
Method 1: Using Object method (easy peasy)
const array1 = ["one", "two", "three", "four", "five"];
const object1 = Object.assign({}, array1);
// Output
console.log(object1);
// Expected Output
// {0: "one", 1: "two", 2: "three", 3: "four", 4: "five"}
Method 2: Using for loop
const array2 = ["apple", "banana", "orange", "grape", "kiwi"];
const object2 = {};
for (let i = 0; i < array2.length; i++) {
object2[i] = array2[i];
}
// Output
console.log(object2);
// Expected Output
// {0: "apple", 1: "banana", 2: "orange", 3: "grape", 4: "kiwi"}
Conclusion:
We have learned about how to convert arrays into objects in JavaScript. We have explore two method to convert the array into object Object.assign() function and for loop method.
I hope it will help you.
Happy Coding :)