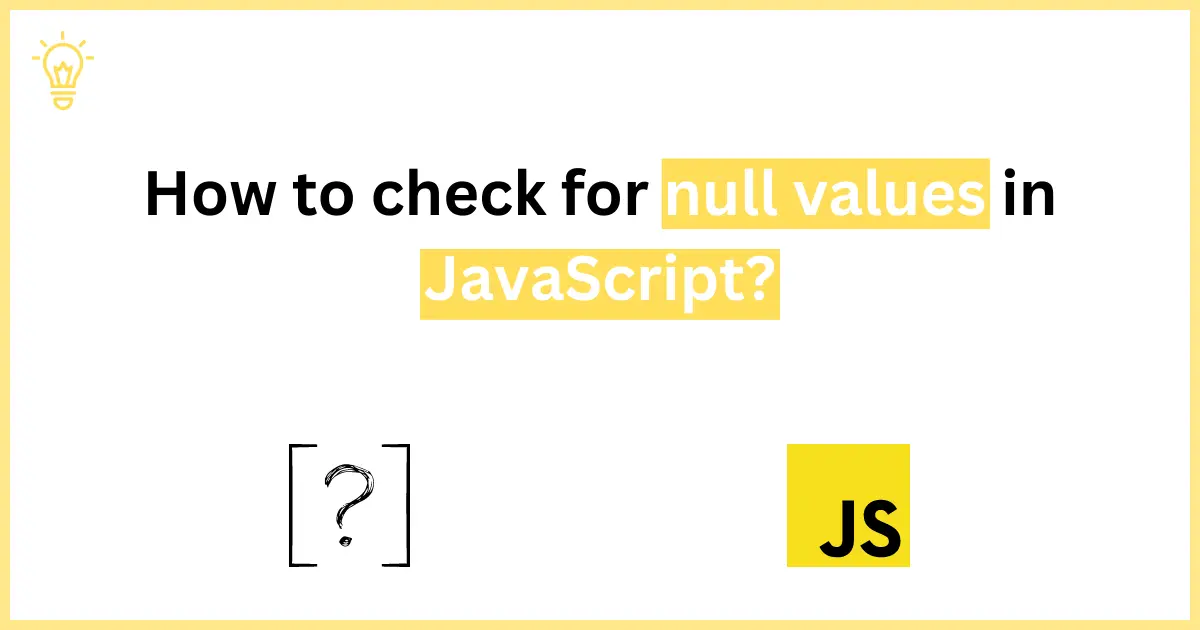
How to check for null values in JavaScript?
Hello Devs,
Sometimes, you need to check for null values in JavaScript to build logic. You can check null values in javascript in different ways. I will show you a few methods to check for null values in JavaScript with examples. We will use javascript equality operators, Object.is() function and typeof() operator in Javascript to check for null values.
Let's first discuss a little about what null is in Javascript before starting to check for null values.
What is null in JavaScript?
Null is a special value in javascript that represents an object that's value is not defined yet. It is a primitive data type that contains the value of a null. Let's see the JavaScript specification that is about null:
null is a primitive value that represents the intentional absence of any object value.
How to Check for Null in JavaScript using Object.is() Function
You can check for null values with Object.is() a built-in function in javascript.
const value = null;
if (Object.is(value, null)) {
console.log("The value is null.");
} else {
console.log("The value is not null.");
}
How to Check for Null in JavaScript with Equality Operators
You can check for null values in JavaScript with equality operators ('==', '==='). Both can be used to check the null values, but use them carefully. They have different behavior in terms of type comparison. Let's have a look at both one by one.
Using the loose equality operator to check for null
In the following code, we are checking null values with the loos equality operator that will consider null and undefined values equal because null and undefined are both false in JavaScript, so the condition will be true.
const nullVal = null;
const unfVal = undefined;
console.log(nullVal == null); // true
console.log(nullVal == unfVal); // true
Using the strict equality operator to check for null
The best way to check for null values in javascript is a strict equality operator. So, the condition will be false.
const nullVal = null;
const unfVal = undefined;
console.log(nullVal === null); // true
console.log(nullVal === unfVal); // false
Read also: How to check if array is empty or null in JavaScript
How to Check for Null with typeof() operator
You can use typeof() operator to check for null values in JavaScript.
const nullVal = null;
const unfVal = undefined;
console.log(typeof(nullVal)); // object
console.log(typeof(unfVal)); // undefined
Wait, did you notice? Why are you getting typeof() object for null values? It is because of a historical bug in JavaScript.
Conclusion:
We have learnt about how to check for null values in JavaScript. I have given you three examples to check for null values in JavaScript. Now you can check for null values with Object.is() method, typeof operator and equality operators in JavaScript.
I hope it will help you.
Happy Coding :)