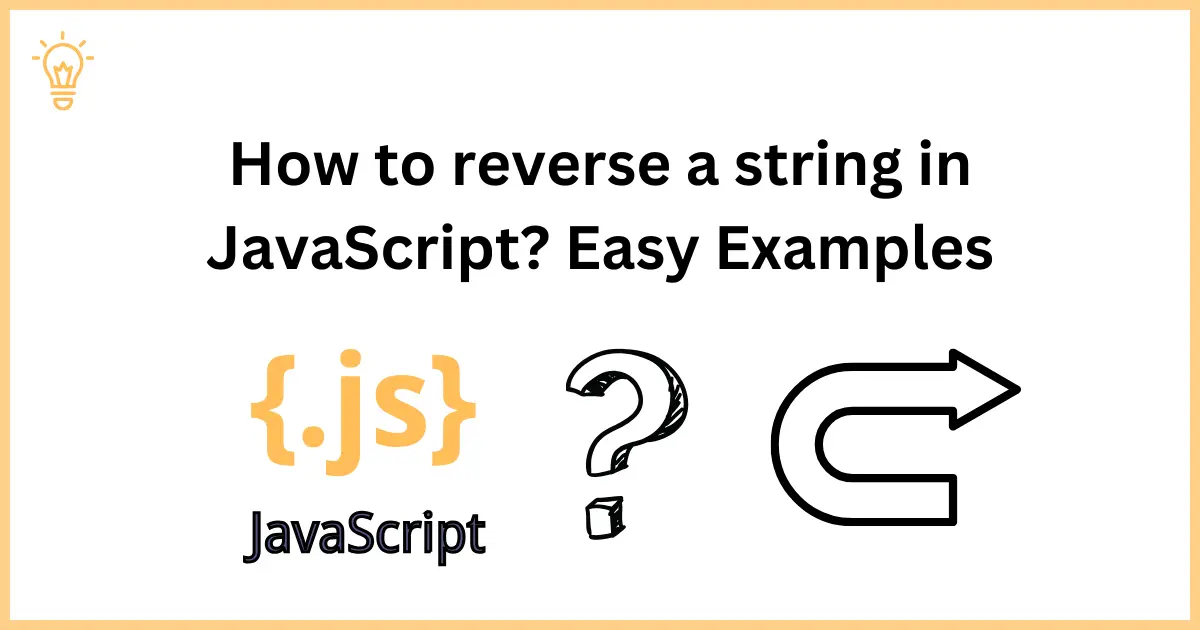
How to reverse a string in JavaScript? Easy Examples
Hello Guys,
Today we are going to learn about how to reverse a string in JavaScript. I will show you a few quick methods to reverse a string in JavaScript. So let's get started without wasting time.
Method 1: Using the reverse() method
In JavaScript, there is no built-in function to reverse a string. We have to use two more built-in functions along with the reverse() function: split() and join(). First, we will split the string with the split() function.
const myString = 'Hello World';
const splitString = myString.split('');
// output: ['H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd']
Now it's time to use the reverse() function to reverse an array.
const splitString = myString.split('');
const reverseString = splitString.reverse();
// output: ['d', 'l', 'r', 'o', 'W', ' ', 'o', 'l', 'l', 'e', 'H']
Now we will use the join() function to convert the array into a string.
const reverseString = splitString.reverse();
cons newString = reverseString.join('');
// output: dlroW olleH
We can create a function as well to make it easy and usable in multiple places in your code. See the following example:
const reverseText = (str) => {
return str.split('').reverse().join('');
}
You can use this function anywhere in your code; you just have to pass your string into the function parameters like this.
const myString = 'Hello World';
reverseText(myString);
// output: dlroW olleH
As you can see, we used the same functionality with a few lines of code to make a reuseable function. Let's explore other methods as well. We will create a reusable function to reverse a string in JavaScript from now on. You just have to update the inner code of the function according to your suitable example.
Method 2: Using the reduce() method
const myString = "Hello World";
function reverseText(str) {
return [...str].reduce((accumulator, current) => current + accumulator)
}
console.log(reverseText(myString ))
// output: dlroW olleH
Method 3: Using the reduceRight() method
const myString = "Hello World";
function reverseString(str) {
return [...str].reduceRight((accumulator, current) => accumulator + current);
}
console.log(reverseText(myString ))
// output: dlroW olleH
Method 4: Using the for loop
const myString = "Hello World";
function reverseString(str) {
let result = "";
for (let i = str.length - 1; i >= 0; i--) {
result += str[i];
}
return result;
}
console.log(reverseText(myString ))
// output: dlroW olleH
Method 5: Using the sort() method
const myString = "Hello World";
function reverseString(str) {
return str
.split("")
.sort(() => -1)
.join("");
}
console.log(reverseText(myString ))
// output: dlroW olleH
Method 6: Using the Recursion Method
const myString = "Hello World";
function reverseString(str = "") {
const [head = "", ...tail] = str;
if (tail.length) {
return reverseString(tail) + head;
}
return head;
}
console.log(reverseText(myString ))
// output: dlroW olleH
Conclusion:
In this tutorial, we have learned about how to reverse a string in JavaScript. We have explored different methods, and we got the same results with all the different methods. You can use any of them according to your needs and logic.
I hope it will help you.
Happy Learning:)