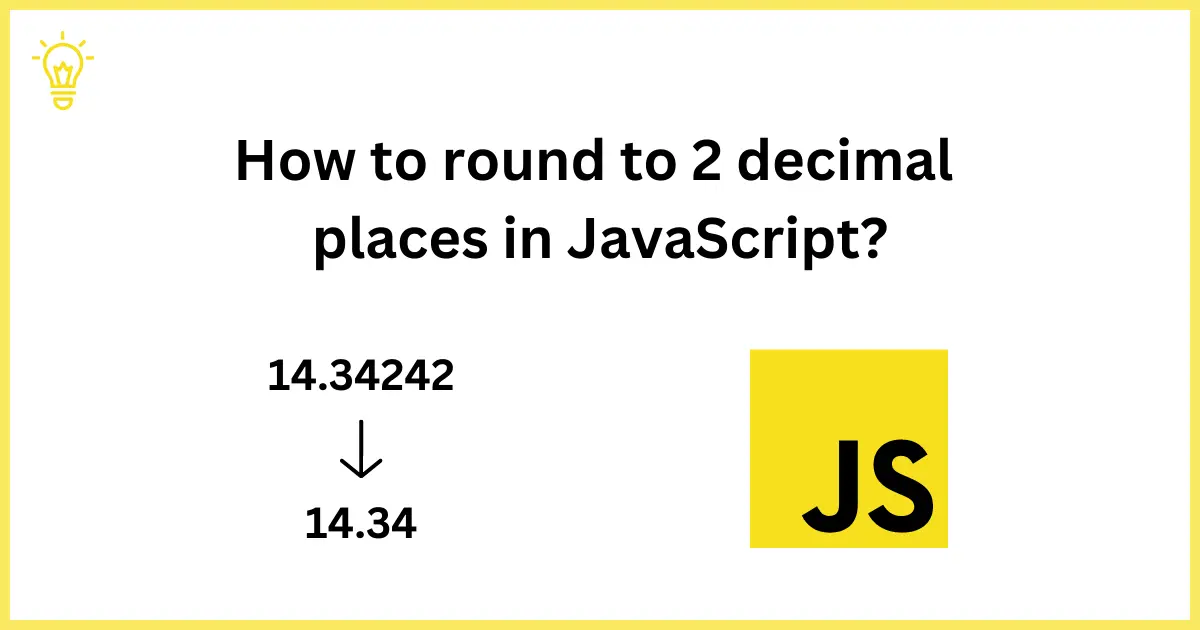
How to round to 2 decimal places in JavaScript?
Hello Devs,
In this tutorial, we will learn about a common task in JavaScript: how to round to two decimal places in JavaScript. Whether you are dealing with price calculations or simply need to display long numbers accurately, We will see the various methods available in JavaScript to achieve this task. Mastring, the art of rounding the numbers, will undoubtedly boost your JavaScript coding skills.
Let's see different methods to round the numbers in JavaScript.
Example 1: Using Math.round() to round to two decimal places in JavaScript
You can use the Math.round() method to round the numbers to 2 decimal places. You need to multiply the number by 100, apply the Math.round() method, and then divide by 100.
var num=14.34242;
var roundedNum=Math.round(num*100)/100;
console.log(roundedNum);
Output
14.34
But this method will not work correctly for all numbers. Let's see this number, 1.005, with an example:
var num=1.005;
var roundedNum=Math.round(num*100)/100;
console.log(roundedNum);
Output
1
But it should be 1.01. To correct this behavior, we can use the number.EPSILON, along with the Math.round method, to a number before multiplying it by 100.
var num=1.005;
var roundedNum=Math.round((num + Number.EPSILON)*100)/100;
console.log(roundedNum);
Output
1.01
Now, you have the correct rounding number in this scenario.
Example 2: Using Number.toFixed() to round to two decimal places
We can use the number.toFixed() method to round the number to 2 decimal places. This method takes a number for which you want to round the number.
var num=1.005;
var roundedNum=num.toFixed(2);
console.log(roundedNum);
Output
1.00
As we can see, this will not work in some cases, as expected. This method will always return 2 decimal places. For example:
- If the input number is 1.1, then it will show the output as 1.10.
- If the input number is 1.156, then it will show the output as 1.15.
Example 3: Using a custom function to round to two decimal places
We can write our own custom function to round the number to 2 decimal places in JavaScript.
function roundNumberTo2Decimal(n) {
return +(Math.round(n + "e+2") + "e-2");
}
console.log(roundNumberTo2Decimal(1.225));
console.log(roundNumberTo2Decimal(1.005));
Output
1.23
1.01
Conclusion:
Rounding the numbers to two decimal places in JavaScript is a common task that can be achieved using different methods. In this tutorial, we have checked three different methods to round the numbers to 2 decimal places in JavaScript. Math.round() and Number.toFixed() methods can be used, but they may not give the expected output in some cases. We can use our custom-generated function as well to round the number in JavaScript.
I hope it will help you.
Happy Coding :)