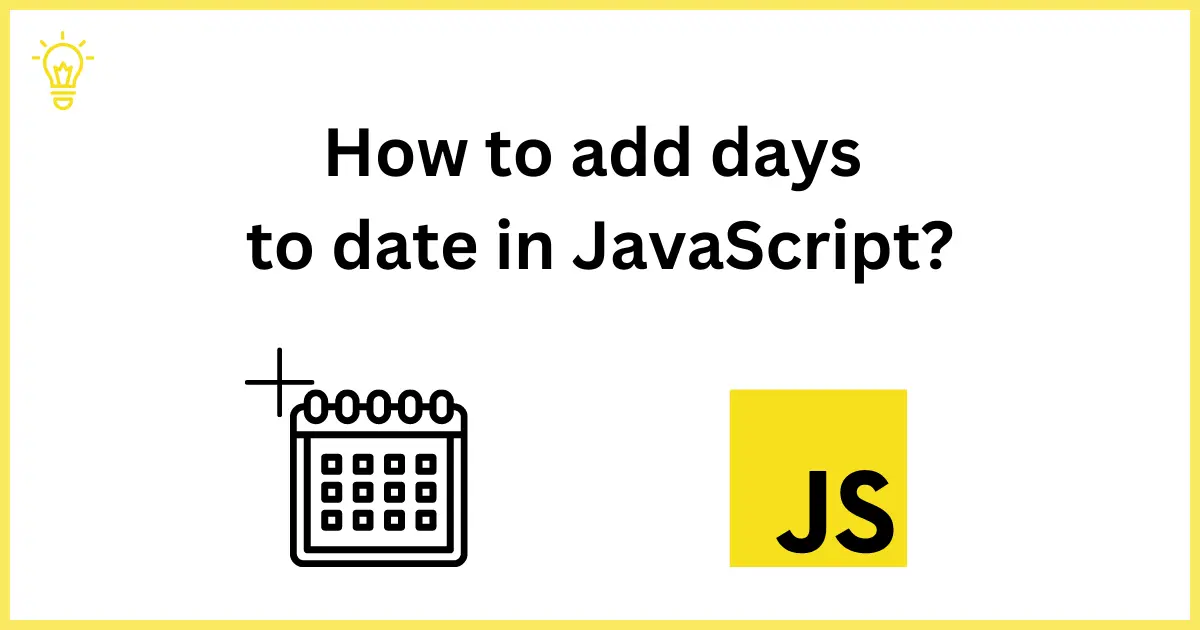
How to add days to date in JavaScript?
Hello Devs,
Today, we will explore how to add days to date in JavaScript. Whether you need to calculate future dates or perform any date operations, you should have to know about how to add days is essential. We will see various methods in JavaScript to achieve this task. After reading this post, you will be able to manipulate dates with ease and add dynamic functionality to your JavaScript application.
Let's see examples now.
Example 1: Date setDate() and getDate() Methods
We can simply use the getDate() and setDate functions to add days to an existing date. We will get the date with the getDate() method, then add the required days and then set the date with the setDate() method. Let's see an example.
const date = new Date();
const newDate = date.getDate() + 7;
date.setDate(newDate)
console.log(date); // "2023-08-16T09:09:38.524Z"
We can create a custom function addDays() to prevent code duplication. This function will take a single parameter for days, then this function will return to a new date with days added. Let's see the following example.
function addDays(days) {
const date = new Date();
date.setDate(date.getDate() + days);
return date;
}
const newDate = addDays(7);
console.log(newDate); // "2023-08-16T09:17:01.074Z"
Example 2: date-fns addDays() method
Alternatively, we can use an npm package date-fns. It provides the pure addDays() function to quickly add the days to a date. You have to install date-fns before to use it. You can simply run the npm i date-fns
command to install it.
import { addDays } from 'date-fns';
const date = new Date();
const newDate = addDays(date, 5);
console.log(newDate); // "2023-08-16T09:17:01.074Z"
Conclusion:
You can use the built-in 'Date' object to add days to a date in JavaScript. By creating a new 'Date' instance with the required date and using the 'setDate()' function, you can increase the date by adding a specified number of days. With the help of the new 'Date' instance and 'setDate()', you can retrieve the incremented date using the 'getDate()' method. You can easily manipulate dates and perform calculations based on the specific requirements in JavaScript.