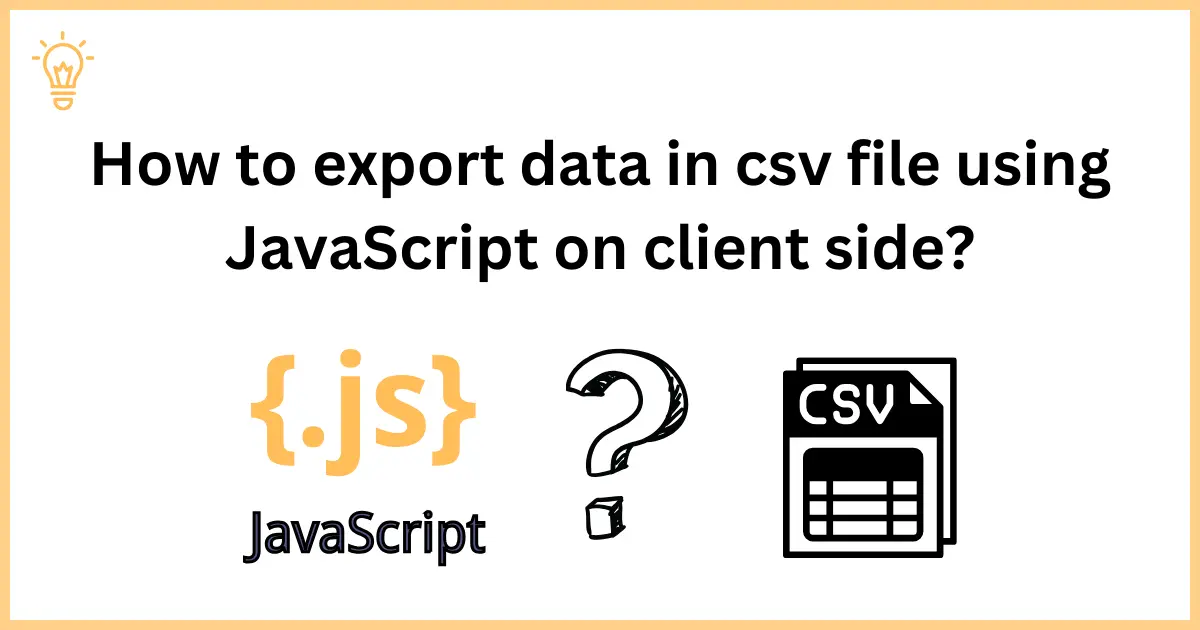
How to export data in csv file using JavaScript on client side?
Have you ever needed to empower your web application users with the ability to export the data into CSV files, enabling them to work with the data in their preferred spreadsheet software? What if I told you that you could do this with just a few lines of JavaScript and jQuery code? Today we will learn about how to export data into a csv file using JavaScript and jQuery on the client side.
Let's get started with the examples.
Export data into CSV files using JavaScript
The following code will show you a simple and quick way to convert JSON data to CSV file using JavaScript. We will use the Blob function to generate the CSV file in JavaScript.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>How to export JSON data into CSV file using JavaScript? - Coders Vibe</title>
</head>
<body>
<h1>How to export JSON data into CSV file using JavaScript? - Coders Vibe</h1>
<a id="createCSVFile" download="file.csv">Download</a>
</body>
<script>
const data = [
{
"id": "1",
"name": "Coders Vibe",
"email": "[email protected]",
},
{
"id": "2",
"name": "JavaScript",
"email": "[email protected]",
},
{
"id": "3",
"name": "jQuery",
"email": "[email protected]",
}
];
const keys = Object.keys(data[0]);
const commaSeparatedString = [keys.join(",") , data.map(row => keys.map(key => row[key]).join(",")).join("\n")].join("\n");
const csvBlob = new Blob([commaSeparatedString]);
const createCSVFile = document.getElementById("createCSVFile");
createCSVFile.href = URL.createObjectURL(csvBlob);
</script>
</html>
Export data into CSV file using jQuery
Using JavaScript is also quite a simple method to export data into CSV file, but if you want to use jQuery, then there it also has a simpler way as well. If your project has jQuery dependencies, then you can use this piece of code. In the following code, we will use a concatenation method along with "data:text/csv;charset=utf-8,"
.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>How to export JSON data into CSV file using jQuery? - Coders Vibe</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"></script>
</head>
<body>
<h1>How to export JSON data into CSV file using jQuery? - Coders Vibe</h1>
<button id="exportButton">Export as CSV</button>
</body>
<script>
$('#exportButton').on('click', function () {
const data = [
{
"id": "1",
"name": "Coders Vibe",
"email": "[email protected]",
},
{
"id": "2",
"name": "JavaScript",
"email": "[email protected]",
},
{
"id": "3",
"name": "jQuery",
"email": "[email protected]",
}
];
var csvContent = "data:text/csv;charset=utf-8,";
var headers = Object.keys(data[0]);
csvContent += headers.join(",") + "\r\n";
data.forEach(function (item) {
var row = [];
headers.forEach(function (header) {
row.push(item[header]);
});
csvContent += row.join(",") + "\r\n";
});
// Create a data URI for the CSV content
var encodedUri = encodeURI(csvContent);
// Create a link to trigger the download
var link = document.createElement("a");
link.setAttribute("href", encodedUri);
link.setAttribute("download", "exported_data.csv");
document.body.appendChild(link);
// Trigger the click event on the link to start the download
link.click();
});
</script>
</html>
We have used JSON data. What if you have data in array format? Don't worry, you can update your code with the following.
-- -- --
data.forEach(function(row) {
var rowContent = row.join(",");
csvContent += rowContent + "\r\n";
});
// Create a data URI for the CSV content
var encodedUri = encodeURI(csvContent);
-- -- -- -
Conclusion
It is a great feature to export data into a csv file on the client side without sending a request to the server. This feature can boost the user experience on your web application. We have covered two methods to export data into CSV file. The one is JavaScript and the other one is jQuery. In the first method, we used vanilla JavaScript and the Blob function to create the CSV file, and in the second method, jQuery comes in handy to export data with a concatenation method.
I hope it will help you.
Happy Learning :)