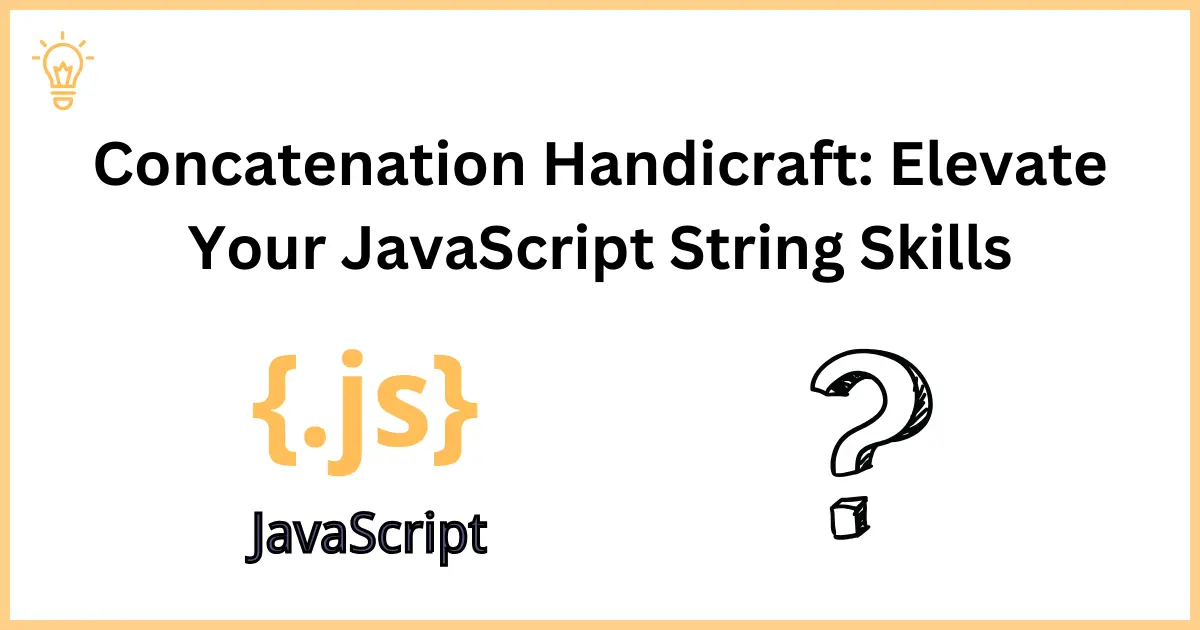
Concatenation Handicraft: Elevate Your JavaScript String Skills
How can you master the art of string concatenation in JavaScript and stay ahead in the ever-evolving web development landscape? This article will delve into various concatenation methods, exploring new language features. Get ready to enhance your string-handling skills and craft more sophisticated and efficient JavaScript code. Whether a novice or a seasoned developer, this guide equips you with the expertise to excel in concatenation and future-proofing your JavaScript endeavours.
5 Basic String Concatenation Examples in JavaScript
Using the + Operator
const str1 = "Hello, ";
const str2 = "world!";
console.log(str1 + str2); // Output: "Hello, world!"
This is the most basic way to concatenate strings in JavaScript. The + operator combines two strings and returns a new string containing both original strings.
Using Template Literal +code + output
const str1 = "Hello, ";
const str2 = "world!";
console.log(`${str1}${str2}`); // Output: "Hello, world!"
Template literals are a more modern way to concatenate strings in JavaScript. They are easier to read and maintain than using the + operator. Template literals allow you to embed expressions inside ropes, which can help create dynamic strings.
Using the Array Join () Methods
const arr = ["Hello", ",", "world", "!"]
console.log(arr.join("")); // Output: "Hello, world!"
The array join() method takes an array of strings and returns a new string that contains all of the original strings joined together by the specified separator. The default separator is a comma, but you can select any character or string as the separator.
Combining Multiple Strings
const str1 = "Hello, ";
const str2 = "world!";
const str3 = "How are you?";
console.log(`${str1}${str2} ${str3}`); // Output: "Hello, world! How are you?"
You can concatenate as many strings as you want using template literals. Simply enclose the strings in curly braces and separate them with spaces or other characters.
Concatenating String and Boolean
const str1 = "Hello, ";
const bool = true;
console.log(`${str1}${bool}`); // Output: "Hello, true"
JavaScript automatically converts the Boolean value to a string before concatenating it with the other series.
All of the above string concatenation examples are still valid in the latest version of JavaScript (ES12). However, a few new features have been introduced in recent versions of JavaScript that can make string concatenation easier and more efficient.
For example, the ES10 String.prototype.concat() method now supports spreading, allowing you to concatenate multiple strings without explicitly typing them out. For example, the following code is equivalent to the previous model:
const str1 = "Hello, ";
const str2 = "world!";
const str3 = "How are you?";
console.log(str1.concat(str2, str3)); // Output: "Hello, world! How are you?"
Another new feature is the ES11 String.prototype.padStart() and String.prototype.padEnd() methods. These methods allow you to pad a string with a specified character or string on the left or right side. This can be useful for creating formatted strings, such as aligning columns in a table.
Overall, string concatenation in JavaScript is a simple task. However, a few new features have been introduced in recent versions of JavaScript that can make string concatenation easier and more efficient.
String Concatenation in ES5 for Legacy Projects
In ES5 for legacy projects, string concatenation can be achieved using the + operator or template literals, ensuring compatibility with older JavaScript versions. While using the + operator, exercise caution when handling special characters like newlines and tabs.
Additionally, you can optimize concatenation tasks by incorporating libraries like lodash for enhanced string manipulation and performance.
Front-end and Back-End Development
String concatenation techniques should remain consistent for code maintainability in front-end or back-end development. However, it's essential to be mindful of distinct environments and constraints in each context, such as client-side browser compatibility or server-side resource utilization.
To bridge the gap between modern and legacy JavaScript, consider using a transpiler to compile current code into ES5, ensuring broad compatibility across various projects.
Best Practices in Modern JavaScript
- Use template literals instead of the + operator for string concatenation.
- Avoid using special characters in strings unless necessary.
- Use functions and variables to make your code more readable and reusable.
Data Visualization
- Use string concatenation to combine different data elements into a single string.
- Use template literals to create dynamic and formatted strings for data visualization.
- Consider using a library to help with data visualization, such as D3.js.
Handling Special Characters in Concatenation
- Escape special characters before concatenating them with other strings.
- Use a regular expression to remove special characters from strings.
- Use a library to help with special character handling, such as lodash.
Conclusion
In the dynamic domain of JavaScript, mastering string concatenation is akin to wielding a potent tool. This article has explored various techniques, from the + operator to template literals, equipping developers with skills to craft efficient code. Whether in legacy or modern projects, the ability to concatenate strings seamlessly is essential. Consider nuances in front-end and back-end development and employ best practices for code clarity. With these insights, you're well-prepared for elegant and data-rich applications in web development's ever-evolving landscape.
Faqs:
What are strings in JavaScript?
Strings in JavaScript: In JavaScript, strings are sequences of characters enclosed in single ('') or double ("") quotes, used to represent and manipulate textual data.
What are template literals in JavaScript, and how can they be used for string concatenation?
Template Literals: Template literals in JavaScript, denoted by backticks (`), allow for easy interpolation and multiline strings. For example, const name = "John"; const greeting = Hello, ${name}!;.
How does string concatenation fit modern web development practices and frameworks?
Modern Web Development: String concatenation, especially with template literals, aligns with current web development practices by enabling dynamic content generation, simplifying code readability, and facilitating integration with popular frameworks like React and Angular.
Is there a limit to the length of concatenated strings in JavaScript?
String Length: There's no strict limit to concatenated string length in JavaScript, but practical limitations like memory availability and browser constraints can affect handling very long strings.
How do I handle variables and expressions within string concatenation in JavaScript?
Handling Variables: JavaScript makes incorporating variables and expressions within string concatenation simple using template literals or the + operator, allowing developers to create dynamic, data-driven content and messages in web applications.