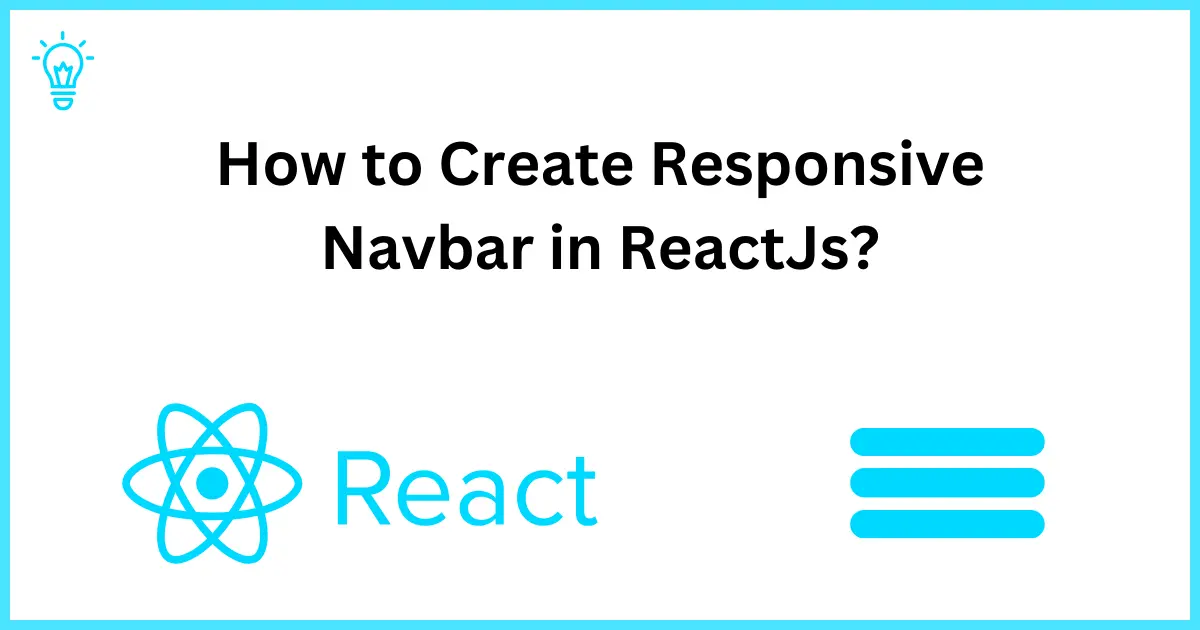
How to Create Responsive Navbar in ReactJs?
Hello Guys,
Whenever you visit any site, the navbar is a focused way to navigate the whole website. So the navbar must be responsive for all devices. Our goal today is to creating a responsive navbar to make it easier for users to navigate websites. Today, we will learn about how to create a responsive navbar in ReactJs and Tailwind CSS.
I hope you have the React application setup to start creating the navigation, but if not, you can follow this blog to setup the React application with Tailwind CSS.
So let's get started with creating a basic structure for our navbar component.
Step 1: Create a Navbar Component
First, you have to create a navbar component.
import { NavLink } from "react-router-dom";
const Navbar = () => {
// Array containing navigation items
const navItems = [
{ id: 1, text: "Home", target: '' },
{ id: 2, text: "Company", target: '/company' },
{ id: 4, text: "About", target: '/about' },
{ id: 5, text: "Contact", target: '/contact' },
];
return (
<nav className="bg-gray-800 p-4">
<div className="flex justify-between items-center max-w-[1200px] mx-auto px-4 text-white">
{/* Logo */}
<NavLink to={''}>
<h1 className="w-full text-3xl font-bold text-white">LOGO.</h1>
</NavLink>
{/* Desktop Navigation */}
<ul className="hidden md:flex">
{navItems.map((item) => (
<li
key={item.id}
className="px-4 py-1 hover:bg-white rounded-xl m-2 cursor-pointer duration-300 hover:text-black"
>
<NavLink to={item.target}>
{item.text}
</NavLink>
</li>
))}
</ul>
</div>
</nav>
);
};
export default Navbar;
As you can see, I have set a max width max-w-[1200px]. It will not exceed 1200 pixels when screen sizes go big.
Quick Question:
Did you notice I'm using NavLink instead of an anchor tag? What will happen if I use an anchor tag? Find the answer at the end of this blog :)
So, I have created an object navItems to define my navbar links, and after that, I have added a map function to my navItems to show nav links. As you can see, I have used Tailwind CSS classes; I have not used any custom CSS till now.
Step 2: Now Make a Responsive Navbar
Now, we will handle the responsiveness of our navbar. Let's update our navbar component.
import React, { useState } from "react";
import { AiOutlineClose, AiOutlineMenu } from "react-icons/ai";
import { NavLink } from "react-router-dom";
const Navbar = () => {
// State to manage the navbar's visibility
const [isNavbarOpen, setIsNavbarOpen] = useState(false);
// Toggle function to handle the navbar's display
const toggleNavbar = () => {
setIsNavbarOpen(!isNavbarOpen);
};
// Array containing navigation items
const navItems = [
{ id: 1, text: "Home", target: '' },
{ id: 2, text: "Company", target: '/company' },
{ id: 4, text: "About", target: '/about' },
{ id: 5, text: "Contact", target: '/contact' },
];
return (
<nav className="bg-gray-800 p-4">
<div className="flex justify-between items-center max-w-[1200px] mx-auto px-4 text-white">
{/* Logo */}
<NavLink to={''}>
<h1 className="w-full text-3xl font-bold text-white">LOGO.</h1>
</NavLink>
{/* Desktop Navigation */}
<ul className="hidden md:flex">
{navItems.map((item) => (
<li
key={item.id}
className="px-4 py-1 hover:bg-white rounded-md m-2 cursor-pointer duration-300 hover:text-black"
>
<NavLink to={item.target}>
{item.text}
</NavLink>
</li>
))}
</ul>
{/* Mobile Navigation Icon */}
<div onClick={toggleNavbar} className="block md:hidden">
{isNavbarOpen ? <AiOutlineClose size={20} /> : <AiOutlineMenu size={20} />}
</div>
{/* Mobile Navigation Menu */}
<ul
className={
isNavbarOpen
? "fixed md:hidden left-0 top-0 w-[60%] h-full border-r border-r-gray-900 bg-[#000300] ease-in-out duration-500"
: "ease-in-out w-[60%] duration-500 fixed top-0 bottom-0 left-[-100%]"
}
>
{/* Mobile Logo */}
<NavLink to={''} className={'ml-3 mt-1 mb-2 block'}>
<h1 className="w-full text-3xl font-bold text-white">LOGO.</h1>
</NavLink>
{/* Mobile Navigation Items */}
{navItems.map((item) => (
<li
key={item.id}
className="text-white hover:bg-gray-700 px-4 py-2 block lg:inline-block"
>
<NavLink to={item.target}>
{item.text}
</NavLink>
</li>
))}
</ul>
</div>
</nav>
);
};
export default Navbar;
I have set a state for toggling the mobile navbar and written a code for the mobile navbar as well.
Here is the complete code for the navbar component.
import React, { useState } from "react";
import { AiOutlineClose, AiOutlineMenu } from "react-icons/ai";
import { NavLink } from "react-router-dom";
const Navbar = () => {
// State to manage the navbar's visibility
const [isNavbarOpen, setIsNavbarOpen] = useState(false);
// Toggle function to handle the navbar's display
const toggleNavbar = () => {
setIsNavbarOpen(!isNavbarOpen);
};
// Array containing navigation items
const navItems = [
{ id: 1, text: "Home", target: '' },
{ id: 2, text: "Company", target: '/company' },
{ id: 4, text: "About", target: '/about' },
{ id: 5, text: "Contact", target: '/contact' },
];
return (
<nav className="bg-gray-800 p-4">
<div className="flex justify-between items-center max-w-[1200px] mx-auto px-4 text-white">
{/* Logo */}
<NavLink to={''}>
<h1 className="w-full text-3xl font-bold text-white">LOGO.</h1>
</NavLink>
{/* Desktop Navigation */}
<ul className="hidden md:flex">
{navItems.map((item) => (
<li
key={item.id}
className="px-4 py-1 hover:bg-white rounded-md m-2 cursor-pointer duration-300 hover:text-black"
>
<NavLink to={item.target}>
{item.text}
</NavLink>
</li>
))}
</ul>
{/* Mobile Navigation Icon */}
<div onClick={toggleNavbar} className="block md:hidden">
{isNavbarOpen ? <AiOutlineClose size={20} /> : <AiOutlineMenu size={20} />}
</div>
{/* Mobile Navigation Menu */}
<ul
className={
isNavbarOpen
? "fixed md:hidden left-0 top-0 w-[60%] h-full border-r border-r-gray-900 bg-[#000300] ease-in-out duration-500"
: "ease-in-out w-[60%] duration-500 fixed top-0 bottom-0 left-[-100%]"
}
>
{/* Mobile Logo */}
<NavLink to={''} className={'ml-3 mt-1 mb-2 block'}>
<h1 className="w-full text-3xl font-bold text-white">LOGO.</h1>
</NavLink>
{/* Mobile Navigation Items */}
{navItems.map((item) => (
<li
key={item.id}
className="text-white hover:bg-gray-700 px-4 py-2 block lg:inline-block"
>
<NavLink to={item.target}>
{item.text}
</NavLink>
</li>
))}
</ul>
</div>
</nav>
);
};
export default Navbar;
Conclusion:
In this blog, we have learned about how to make a responsive navbar in ReactJS. I have used ReactJs and TailwindCSS to make a responsive navbar. We don't have to use any custom CSS to style the navbar or make it responsive; Tailwind CSS will handle all this.
I hope it will help you make a responsive navbar for your next project.
FAQs
What if you don't use Navlink instead of the anchor (<a>) tag?
If we don't use Navlink, the anchor tag will refresh the page when clicked, which will break the main reason to use ReactJS. Reatjs is used to create a single-page application, so we use Navlink to navigate between the pages.