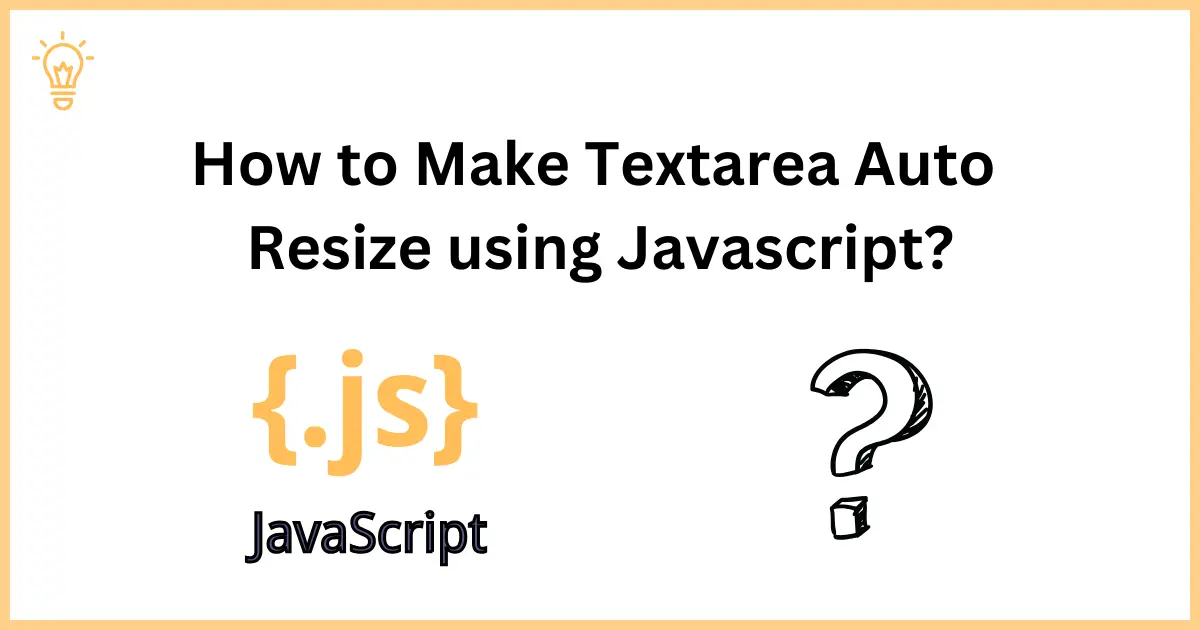
How to Make Textarea Auto Resize using JavaScript?
Have you ever needed how to create a auto growing and user-friendly text input experience where the text-area expands and shrinks as your users type, eliminating the need for endless scrolling? If yes, then go ahead, because we are discussing it today! You will learn about how to make textarea auto resizeable using JavaScript and jQuery. I will show you examples of both Vanilla JavaScript and jQuery. You can use it according to your needs.
So let's get started with the examples.
Auto-growing Textarea with Javascript
<!DOCTYPE html>
<html>
<head>
<title>Javascript Auto-resize Textarea as User Types</title>
</head>
<body>
<h1>Javascript Auto-resize Textarea as User Types - Coders Vibe</h1>
<strong>Message:</strong>
<br/>
<textarea style="width: 400px;"></textarea>
<script>
const textarea = document.querySelector("textarea");
textarea.addEventListener('input', autoResize, false);
function autoResize() {
this.style.height = 'auto';
this.style.height = this.scrollHeight + 'px';
}
</script>
</body>
</html>
Auto Resize Textarea with jQuery
<!DOCTYPE html>
<html>
<head>
<title>Textarea Auto Height Based on Content Jquery</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"></script>
</head>
<body>
<h1>Textarea Auto Height Based on Content Jquery - Coders Vibe</h1>
<strong>Body:</strong>
<br/>
<textarea style="width: 400px;"></textarea>
<script type="text/javascript">
$('textarea').on('input', function () {
this.style.height = 'auto';
this.style.height = (this.scrollHeight) + 'px';
});
</script>
</body>
</html>
Custom AutoResizeTextarea Function
If you have to implement it in multiple places in your project, then it is good to use a functional approach to prevent rewriting the same code many times.
<!DOCTYPE html>
<html>
<head>
<title>Textarea Auto Height Based on Content Custom function</title>
<script src="https://code.jquery.com/jquery-3.7.1.min.js"></script>
</head>
<body>
<h1>Textarea Auto Height Based on Content custom function Example - Coders Vibe.com</h1>
<strong>Body:</strong>
<br />
<textarea data-autoresize rows="2"></textarea>
<textarea data-autoresize rows="2"></textarea>
<script>
function AutoResizeTextarea() {
document.querySelectorAll('[data-autoresize]').forEach(function(element) {
element.style.boxSizing = 'border-box';
var offset = element.offsetHeight - element.clientHeight;
element.addEventListener('input', function(event) {
event.target.style.height = 'auto';
event.target.style.height = event.target.scrollHeight + offset + 'px';
});
element.removeAttribute('data-autoresize');
});
}
</script>
</body>
</html>
You have to just call the function and it will implement the auto-resize textarea to fit content which has the data-autoresize attribute.
Conclusion:
In conclusion, making textarea auto resizable using JavaScript or jQuery is a good and user-friendly feature that can greatly boost the user experience on your website. In this article, we explored two different methods to make textarea auto-resizealbe. One is using vanilla JavaScript and the other uses jQuery. You are free to choose the method that best suits your project's requirements.
I hope it will help you.
Happy Learning :)