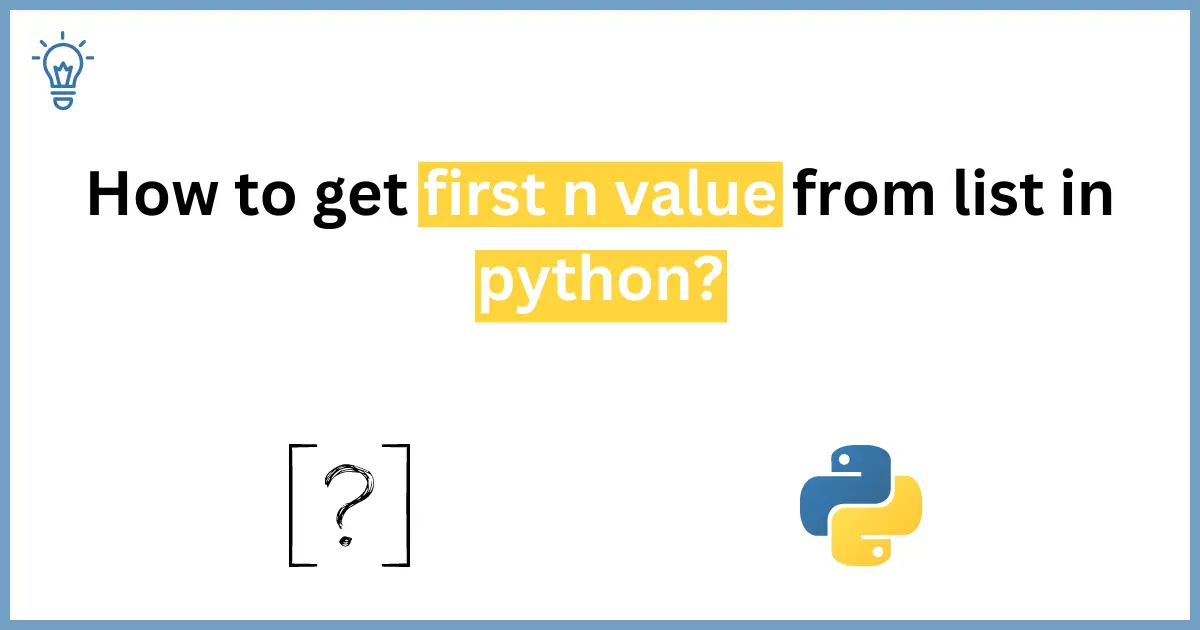
How to get first n value from list in python?
If you have ever come into this scenario where you want to fetch the first N items from a list in python and wonder how to do this, we will explore a simple yet powerful technique that allows you to extract a specific value from the list quickly. Whether you are an absolute beginner or an experienced python developer, mastering this skill will make your workflow smooth. So, let's dive in and discover how to get the first Nth items from a list in python?
Get the First N Elements from the list using Slicing
Slicing is a powerful and easy method to extract specific value from a list in python. We will discuss two slicing methods one by one to get value from a list.
Using List Slicing
Let's say we have a list called "my_list" and we want to retrieve the first N value from "my_list":
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
N = 3 # Number of elements to fetch
first_N_elements = my_list[:N]
Using Slice Function
We can implement the same scenario with the slice function. Let's use our previously defined list "my_list" to get values from "my_list" with a slice function.
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
N = 3 # Number of elements to fetch
sliced_elements = my_list[slice(N)]
Using Loops in Python
To extract the first N value from a list in Python using loops, we can employ a loop structure such as a for loop or a while loop. We will discuss both loops one by one.
Get the First N Elements from the List Using For Loop
Here's an example using a for loop:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
N = 3 # Number of elements to fetch
n_elements = []
for i in range(N):
n_elements.append(my_list[i])
Get the First N Elements from the List Using While Loop
You can also achieve the same result using a while loop:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
N = 3 # Number of elements to fetch
n_elements = []
count = 0
while count < N:
n_elements.append(my_list[count])
count += 1
Get the First N Elements from the List Using List Comprehension
We can use a concise and powerful method to extract the specific value from the list using list comprehension. This method allows us to create a new list by iterating over an existing list and allowing us to apply a condition as well. Let's see an example of how to use list comprehension to get first n value:
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
N = 3 # Number of elements to fetch
first_N_elements = [my_list[i] for i in range(N)]
Using the Itertools Module
We can make use of the islice() function to get first n value from a list in python using the itertools module. This function provides a convenient and quick way to extract a specific number of value from an iterable. Let's see the following example:
importing tools
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
N = 3 # Number of elements to fetch
n_elements = list(itertools.islice(my_list, N))
Conclusion
In conclusion, we can fetch first n value from a list in python through various methods. We have discussed almost 6 different methods in a blog post to get n value from a list in python. At the end of this tutorial, I hope you can use the best option in your next project.
Happy Coding :)