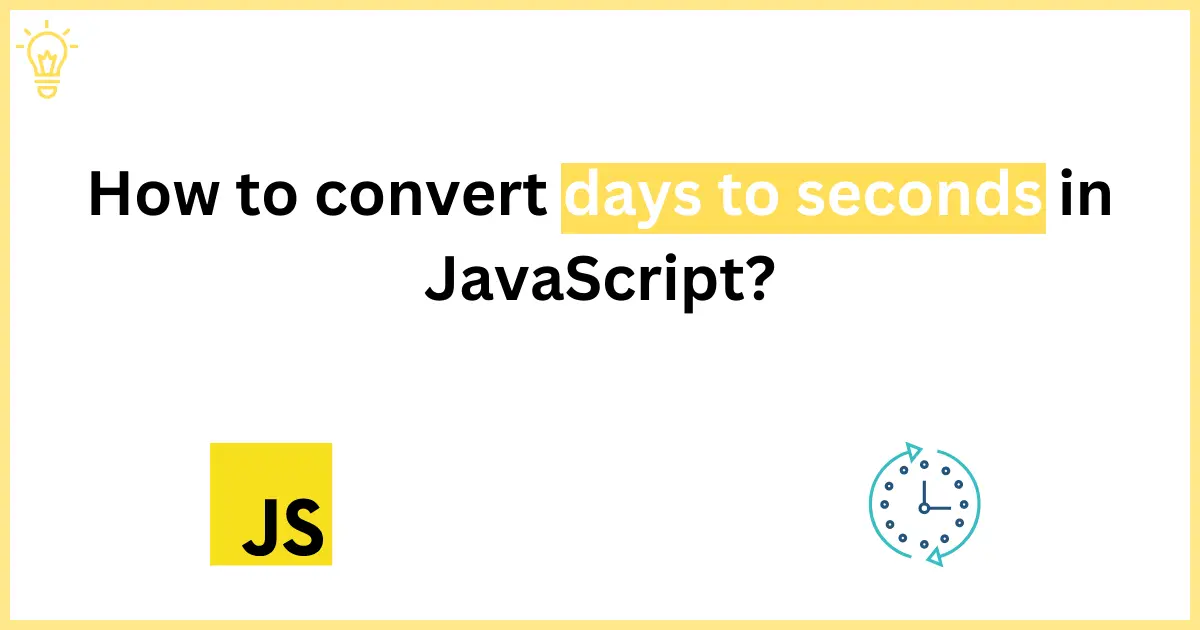
How to convert days to seconds in JavaScript?
Hello Guys,
Today, we will learn how to convert days to seconds in JavaScript. I will show you a few examples of converting days to milliseconds, days to seconds and days to minutes. This tutorial goes into detail, how to convert days to seconds in JavaScript.
So let's get started.
Example 1: Convert Days to Milliseconds in JavaScript
To convert days to milliseconds, we will multiply the days by 24 for the hours, 60 for the minutes, 60 for the seconds and 1000 for the milliseconds. The multiplication will return milliseconds, which will be equivalent to the number of days. See the example below.
function days_to_milliseconds(days) {
// 👇️ hour min sec ms
return days * 24 * 60 * 60 * 1000;
}
// output
console.log(days_to_milliseconds(1)); // 86400000
console.log(days_to_milliseconds(2)); // 17280000
console.log(days_to_milliseconds(5)); // 432000000
As you can see, we have created a reusable function named days_to_milliseconds() that takes days as a parameter and returns milliseconds after converting the days into milliseconds.
Example 2: Convert Days to Seconds using JavaScript
To convert days to seconds, we will multiply the days by 24 for the hours, 60 for the minutes and 60 for the seconds. The multiplication will return seconds, which will be equivalent to the number of days. See the example below.
function days_to_seconds(days) {
// 👇️ hour min sec
return days * 24 * 60 * 60;
}
// output
console.log(days_to_seconds(1)); // 86400
console.log(days_to_seconds(2)); // 172800
console.log(days_to_seconds(5)); // 432000
As you can see, we have created a reusable function named days_to_seconds() that takes days as a parameter and returns seconds after converting the days into seconds.
Example 3: Convert Days to Minutes using JavaScript
To convert days to minutes, we will multiply the days by 24 for the hours and 60 for the minutes. The multiplication will return the minutes, which will be equivalent to the number of days. See the example below. If your application uses fractional units for the number of days, you might get the decimal number after the conversion of days into milliseconds, just like below.
console.log(days_to_milliseconds(5.5612)); // 442367999.99999
So, you can use the Math.round() function to round the number to the nearest integer.
function days_to_milliseconds(days) {
// hour min sec ms
return Math.round(days * 24 * 60 * 60 * 1000);
}
// output
console.log(days_to_milliseconds(5.5612)); // 442368000
Conclusion:
I have shown you three examples to convert days into milliseconds, seconds and minutes. We have simply created a reusable function to do conversions and we have learnt how you can round the numbers if our application uses fractional units for the number of days.
I hope it will help you.
Happy Coding :)